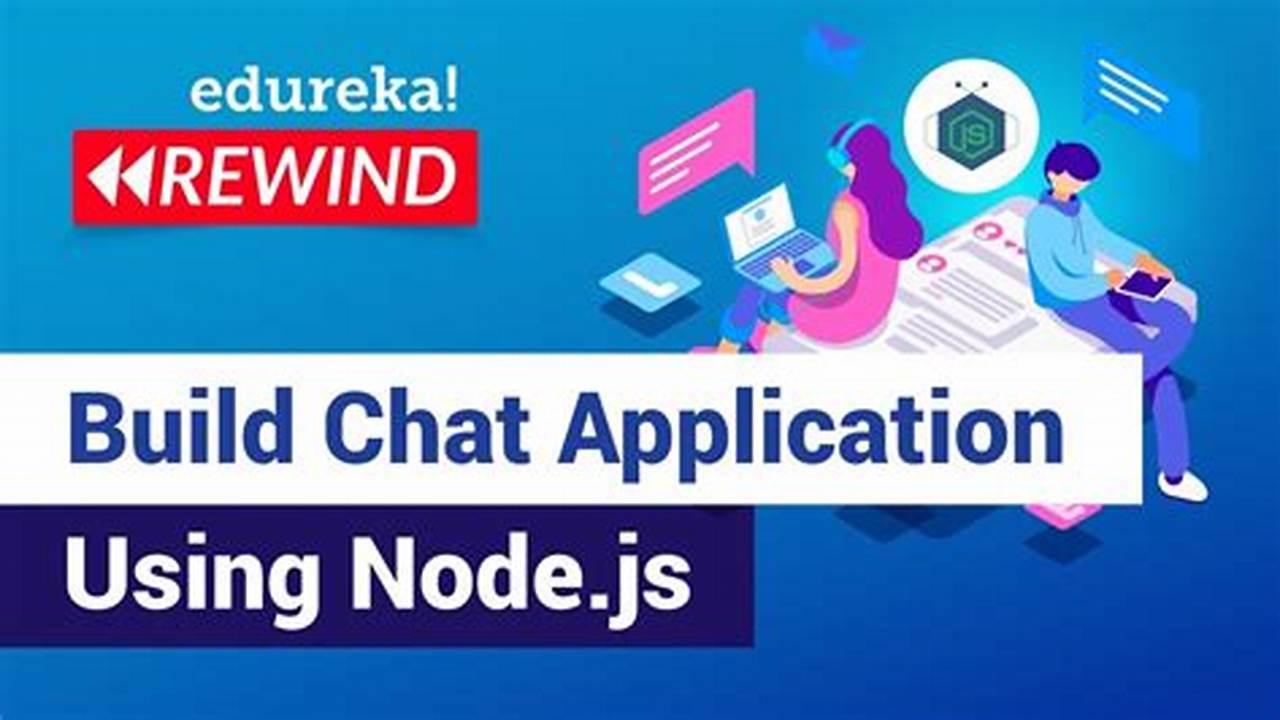
A step-by-step tutorial for building a chat application using Node.js and Socket.IO provides a comprehensive guide on how to create a real-time chat application from scratch. This tutorial is beneficial for developers who want to learn how to build interactive and scalable web applications.
Node.js is a popular JavaScript runtime environment that is well-suited for building real-time applications. Socket.IO is a library that enables real-time communication between a server and multiple clients. By combining Node.js and Socket.IO, developers can create chat applications that are both efficient and easy to use.
This tutorial will cover the following topics:
- Installing Node.js and Socket.IO
- Creating a Node.js server
- Setting up Socket.IO
- Handling client connections
- Sending and receiving messages
- Styling the chat application
step by step tutorial for building a chat application using node js and socket io
A step-by-step tutorial for building a chat application using Node.js and Socket.IO provides valuable guidance to developers of all levels. It covers essential aspects such as:
- Node.js installation
- Socket.IO setup
- Server creation
- Client connection handling
- Message sending and receiving
- Styling techniques
- Code examples
- Real-world use cases
- Troubleshooting tips
These aspects are crucial for building robust and interactive chat applications. They provide a comprehensive understanding of the development process, from setup to deployment. Whether you’re a beginner or an experienced developer, a step-by-step tutorial can empower you to create efficient and scalable chat applications.
Node.js installation
Node.js installation is a crucial step in the process of building a chat application using Node.js and Socket.IO. Node.js is a JavaScript runtime environment that provides the necessary infrastructure for real-time communication. Installing Node.js ensures that your system has the required platform to run the chat application.
-
Prerequisites
Before installing Node.js, ensure that your system meets the minimum requirements, including a recent version of your operating system and sufficient disk space.
-
Installation methods
Node.js can be installed using various methods, including package managers (e.g., npm, yarn), binary installers, and source code compilation. Choose the method that best suits your system and preferences.
-
Environment variables
After installation, set up the necessary environment variables, such as the NODE_PATH and PATH variables, to ensure that the Node.js executables are accessible from the command line.
-
Version management
If you plan to work on multiple projects with different Node.js versions, consider using a version manager like nvm or n to easily switch between versions.
Proper Node.js installation lays the foundation for a successful chat application development process. It ensures that the necessary runtime environment is available and configured correctly, allowing for smooth execution and real-time communication features.
Socket.IO setup
Socket.IO setup is an integral part of building a chat application using Node.js and Socket.IO. Socket.IO is a real-time communication library that enables bi-directional communication between the server and multiple clients connected to it. It provides an abstraction layer over the complex underlying protocols such as WebSockets and HTTP long-polling, allowing developers to focus on building the application logic without worrying about the low-level details of real-time communication.
In a step-by-step tutorial for building a chat application, Socket.IO setup typically involves the following steps:
- Install the Socket.IO package: Using a package manager like npm or yarn, install the Socket.IO package on both the server and client sides.
- Configure the Socket.IO server: On the server side, configure the Socket.IO server to listen for incoming client connections and handle real-time communication.
- Connect to the Socket.IO server: On the client side, establish a connection to the Socket.IO server using the Socket.IO client library.
- Handle events and data exchange: Implement event handlers on both the server and client sides to handle incoming events and exchange data in real-time.
Proper Socket.IO setup ensures that the chat application can establish and maintain real-time connections between multiple clients and the server. It enables real-time data exchange, making it possible for users to send and receive messages, updates, and other data in a seamless and interactive manner.
Server creation
In the context of building a chat application using Node.js and Socket.IO, server creation is a fundamental step that lays the foundation for real-time communication and data exchange. The server acts as a central hub, responsible for managing client connections, facilitating message routing, and maintaining the overall state of the chat application.
Server creation involves setting up a Node.js server using frameworks like Express.js or Fastify.js. The server listens for incoming client connections on a specified port and establishes a persistent connection with each connected client. Once a client connects, the server can send and receive data in real-time, enabling interactive communication between multiple clients.
Server creation is a critical component of a step-by-step tutorial for building a chat application using Node.js and Socket.IO. It provides a comprehensive understanding of how to set up the server-side infrastructure, handle client connections, and manage real-time communication. By understanding the principles of server creation, developers can build robust and scalable chat applications that can handle multiple concurrent connections and data exchanges.
Client connection handling
Client connection handling is a crucial aspect of building a chat application using Node.js and Socket.IO. It involves managing the establishment, maintenance, and termination of connections between clients and the server. Proper client connection handling ensures that clients can connect, send and receive messages, and disconnect gracefully, enabling real-time communication and a smooth user experience.
- Client Authentication: Verifying the identity of connecting clients is essential to prevent unauthorized access and maintain the integrity of the chat application. Authentication mechanisms like token-based authorization or OAuth can be implemented to ensure that only authorized clients can connect.
- Connection Management: Keeping track of active client connections is necessary for efficient communication and resource allocation. Connection management involves maintaining a list of connected clients, monitoring their activity, and handling connection termination gracefully to avoid orphaned connections.
- Data Exchange: Client connection handling involves establishing a reliable channel for data exchange between clients and the server. This includes handling incoming messages, broadcasting messages to all connected clients, and managing message queues to ensure delivery.
- Error Handling: Robust client connection handling should include mechanisms to handle errors and unexpected disconnections. Error handling involves detecting and recovering from network issues, connection timeouts, and other exceptional conditions to maintain application stability and user experience.
Effective client connection handling is key to building scalable and reliable chat applications. By understanding and implementing these aspects, developers can ensure that clients can connect, communicate, and disconnect seamlessly, enhancing the overall user experience and the quality of real-time communication.
Message sending and receiving
In the context of building a chat application using Node.js and Socket.IO, message sending and receiving is a core feature that enables real-time communication between users. It involves the exchange of messages between the client and the server, ensuring that messages are delivered in a timely and reliable manner.
- Message Format: Defining the format of messages is essential for ensuring compatibility between the client and the server. This includes specifying the structure, encoding, and any metadata associated with the messages.
- Message Routing: When multiple clients are connected to the chat application, it’s crucial to implement a mechanism for routing messages to the intended recipients. This involves identifying the target recipient(s) and ensuring that messages are delivered to the correct clients.
- Message Delivery: Reliable message delivery is essential to maintain the integrity of real-time communication. This involves implementing mechanisms for acknowledging received messages, handling message retransmissions, and managing message queues.
- Message Security: In some scenarios, it may be necessary to implement security measures to protect the confidentiality and integrity of messages. This can involve encrypting messages, implementing authentication mechanisms, and managing access control.
Effective message sending and receiving is crucial for building robust and scalable chat applications. By understanding and implementing these aspects, developers can ensure that messages are exchanged efficiently, reliably, and securely, enhancing the overall user experience and the quality of real-time communication.
Styling techniques
Styling techniques play a critical role in the development of a step-by-step tutorial for building a chat application using Node.js and Socket.IO. By incorporating visually appealing and user-friendly design elements, styling enhances the overall user experience and makes the tutorial more engaging and accessible.
Effective styling techniques can improve the readability and organization of the tutorial. Clear and concise text formatting, such as headings, subheadings, and bullet points, helps readers easily navigate and grasp the key concepts. Additionally, the use of color, typography, and white space can create a visually appealing and professional-looking tutorial that is more likely to capture and retain the attention of readers.
Beyond aesthetics, styling techniques can also impact the effectiveness of the tutorial. For example, the use of interactive elements, such as code snippets, live demos, and interactive exercises, can reinforce the learning process and make the tutorial more hands-on and engaging. By incorporating these styling techniques, developers can create a comprehensive and visually appealing tutorial that maximizes the learning experience for users.
Code examples
In the context of a step-by-step tutorial for building a chat application using Node.js and Socket.IO, code examples play a pivotal role in enhancing the learning experience for users. They serve as practical illustrations of the concepts and techniques discussed in the tutorial, enabling readers to apply their knowledge and gain a deeper understanding of the development process.
Code examples are critical components of an effective tutorial, as they provide a hands-on approach to learning. By examining real-life examples of code implementation, readers can grasp the practical application of the concepts and identify potential pitfalls. Furthermore, code examples allow readers to experiment and modify the code to suit their specific needs, promoting a deeper level of engagement and understanding.
For instance, in a section on establishing a Socket.IO connection, a code example would demonstrate how to create a client instance, connect to the server, and handle incoming and outgoing events. This allows readers to visualize the code implementation and replicate the process in their own projects. By providing a concrete reference, code examples accelerate the learning process and make the tutorial more accessible to a wider range of learners.
In summary, code examples are invaluable assets in a step-by-step tutorial for building a chat application using Node.js and Socket.IO. They provide practical guidance, enhance understanding, and promote experimentation, making the learning experience more effective and engaging for users.
Real-world use cases
Real-world use cases play a critical role in the context of a step-by-step tutorial for building a chat application using Node.js and Socket.IO. These use cases provide practical examples of how the concepts and techniques discussed in the tutorial can be applied to solve real-world problems and create valuable applications.
By exploring real-world use cases, learners can gain a deeper understanding of the purpose and significance of the tutorial content. These use cases demonstrate how the underlying technologies can be utilized to address specific communication needs and challenges in various domains.
For instance, a use case might showcase how to build a real-time chat application for customer support, enabling businesses to provide instant and personalized assistance to their customers. Another use case could demonstrate how to create a collaborative online whiteboard using Socket.IO, allowing multiple users to draw and interact with the whiteboard simultaneously.
By incorporating real-world use cases into the tutorial, learners can appreciate the practical value of the concepts they are studying. These use cases help bridge the gap between theory and practice, fostering a more comprehensive and meaningful learning experience.
Troubleshooting tips
Troubleshooting tips play a crucial role in the context of a step-by-step tutorial for building a chat application using Node.js and Socket.IO. These tips provide guidance on how to identify and resolve common issues that may arise during the development process, ensuring a smoother and more successful learning experience.
Troubleshooting tips are indispensable for helping learners anticipate and overcome potential challenges. By providing practical advice on debugging techniques, error handling, and performance optimization, these tips empower learners to troubleshoot issues effectively and independently. This not only deepens their understanding of the underlying technologies but also fosters their problem-solving abilities.
For instance, a troubleshooting tip might suggest using logging statements to identify the source of an error, or it might provide guidance on how to handle disconnections and reconnections gracefully. Real-life examples of troubleshooting tips within a step-by-step tutorial could include resolving issues with message delivery, optimizing server performance under load, or debugging cross-browser compatibility issues.
Understanding the connection between troubleshooting tips and a step-by-step tutorial for building a chat application using Node.js and Socket.IO is essential for learners to develop a comprehensive understanding of the development process. By incorporating troubleshooting tips, learners gain valuable insights into debugging techniques, error handling, and performance optimization, enabling them to build robust and reliable chat applications.
Frequently Asked Questions
This FAQ section provides answers to common questions and clarifies key concepts related to the step-by-step tutorial for building a chat application using Node.js and Socket.IO.
Question 1: What are the prerequisites for building a chat application using Node.js and Socket.IO?
You will need a basic understanding of Node.js, JavaScript, and HTML/CSS. Additionally, you should have a development environment set up with Node.js and a code editor.
Question 2: What are the benefits of using Socket.IO for real-time communication?
Socket.IO provides a seamless and efficient way to establish real-time connections between clients and servers. It handles the complexities of low-level protocols, making it easier to build real-time applications.
Question 3: How do I handle user authentication and authorization in my chat application?
Implementing user authentication and authorization is crucial for securing your chat application. You can use third-party authentication services or create your own custom authentication system using Node.js and a database.
Question 4: What are the best practices for optimizing the performance of a chat application?
To optimize performance, consider using efficient data structures, caching mechanisms, and load balancing techniques. Additionally, optimizing your database queries and minimizing unnecessary network requests can improve responsiveness.
Question 5: How can I deploy my chat application to a production environment?
For deployment, you can use cloud hosting platforms like Heroku or AWS. Ensure you have a proper deployment strategy in place to handle scaling, monitoring, and maintenance.
Question 6: Are there any security considerations I should be aware of when building a chat application?
Security is paramount. Implement measures like encryption, input validation, and rate limiting to protect your application from vulnerabilities and malicious attacks.
These FAQs provide a concise overview of some key aspects of building a chat application using Node.js and Socket.IO. For further in-depth discussion and code examples, refer to the comprehensive tutorial provided.
In the next section, we will explore advanced topics, including integrating additional features and scaling your chat application for larger user bases.
Tips for Building a Robust Chat Application with Node.js and Socket.IO
This section provides a set of practical tips to enhance the development and functionality of your chat application using Node.js and Socket.IO.
Tip 1: Optimize Data Structures: Utilize efficient data structures like hash tables or trees to improve data retrieval and storage, enhancing the performance of your application.
Tip 2: Leverage Caching Mechanisms: Implement caching techniques to store frequently accessed data in memory, minimizing database queries and reducing server load.
Tip 3: Implement Load Balancing: Distribute user requests across multiple servers to handle high traffic and improve scalability, ensuring a smooth user experience.
Tip 4: Minimize Network Requests: Optimize your application by combining multiple requests into a single request or using web sockets to reduce network overhead and improve responsiveness.
Tip 5: Enhance Security Measures: Prioritize security by implementing encryption algorithms, input validation, and rate limiting to protect user data and prevent malicious attacks.
Tip 6: Utilize Scalable Database Solutions: Choose a database that can handle the increasing data demands as your chat application grows, ensuring efficient data management and performance.
Tip 7: Employ Real-Time Analytics: Integrate real-time analytics tools to monitor user behavior, identify usage patterns, and make data-driven decisions to improve the application.
Tip 8: Consider Cross-Platform Compatibility: Ensure your chat application is compatible with different devices and operating systems by implementing responsive design and testing across multiple platforms.
By following these tips, you can build a robust, scalable, and user-friendly chat application that meets the demands of your users and provides an optimal communication experience.
In the concluding section, we will discuss best practices for deploying and maintaining your chat application, ensuring its stability, security, and ongoing success.
Conclusion
This article has provided a comprehensive exploration of “step by step tutorial for building a chat application using Node.js and Socket.IO,” delving into its key concepts, best practices, and advanced techniques.
Key takeaways include the importance of optimizing data structures and leveraging caching mechanisms to enhance performance, implementing load balancing and minimizing network requests for scalability, and prioritizing security measures to protect user data and prevent malicious attacks. Additionally, the exploration of real-time analytics, cross-platform compatibility, and scalable database solutions provides valuable insights into building robust and user-friendly chat applications.
As the demand for real-time communication continues to grow, mastering the art of building chat applications using Node.js and Socket.IO becomes increasingly important. Embracing these best practices and continually seeking knowledge will enable developers to create innovative and scalable solutions that meet the evolving needs of users.
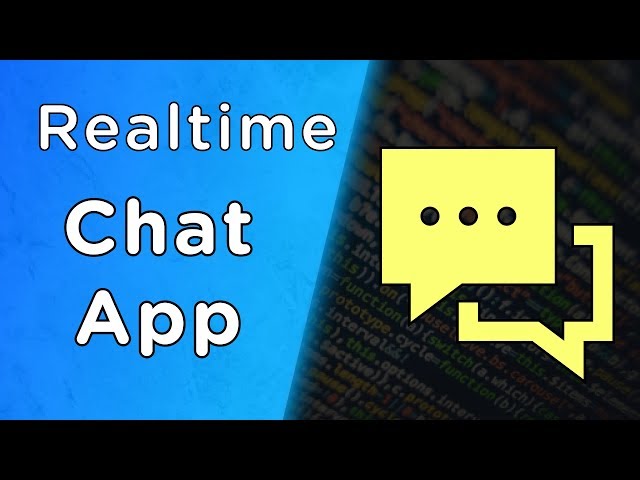