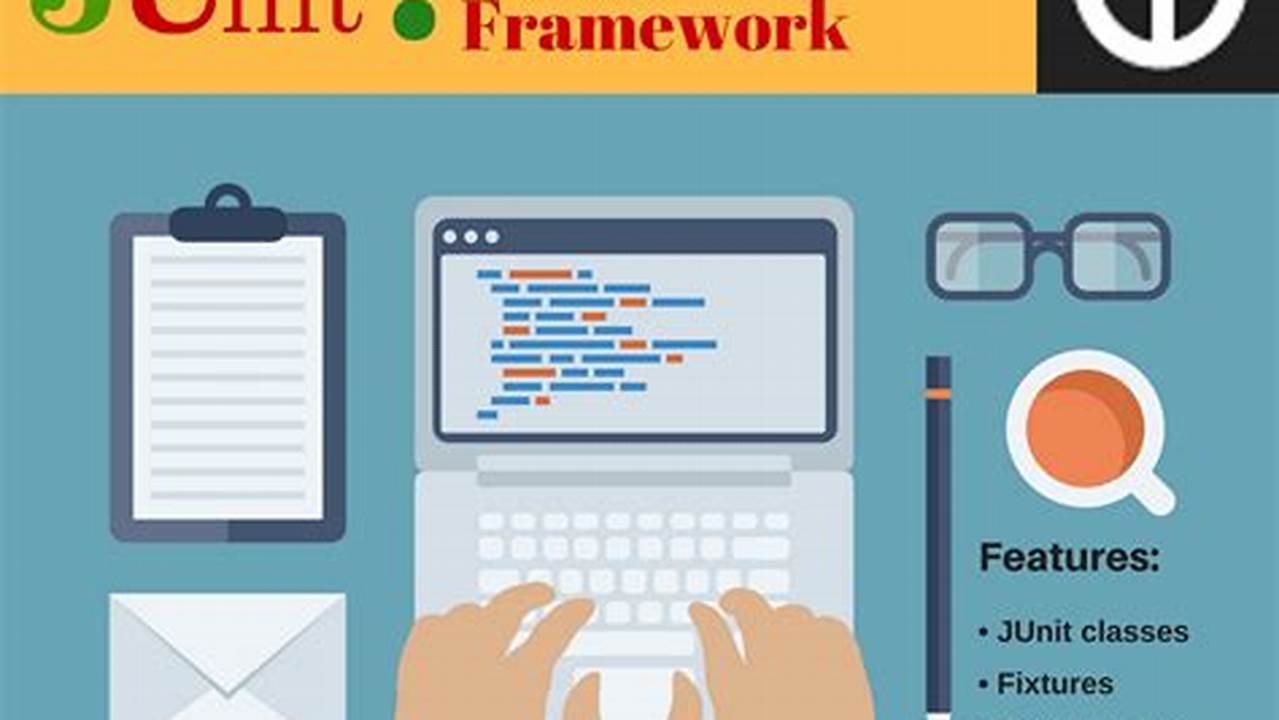
Implementing Unit Testing Frameworks: A Key to Enhanced Code Quality
Implementing unit testing frameworks like JUnit or Pytest is crucial for ensuring the reliability and maintainability of software. These frameworks allow developers to test individual components of their code in isolation, enabling them to quickly identify and fix any issues before they become major problems.
Unit testing has become an essential practice in modern software development, helping to prevent defects and reduce the cost of maintenance. One of the key historical developments in unit testing was the introduction of the JUnit framework in the late 1990s, which provided a standardized and extensible platform for testing Java code.
This article will delve into the benefits of implementing unit testing frameworks, explore advanced testing techniques, and provide practical guidance on how to incorporate unit testing into your development process.
Implementing Unit Testing Frameworks
Implementing unit testing frameworks like JUnit or Pytest is essential for improving the quality of software code. Here are 9 key aspects to consider when implementing unit testing frameworks:
- Modularity: Break down code into smaller, testable units.
- Coverage: Ensure that all code paths are tested.
- Isolation: Test individual units in isolation to avoid dependencies.
- Automation: Automate tests to reduce manual effort and improve efficiency.
- Feedback: Provide immediate feedback on test results to developers.
- Maintainability: Keep tests maintainable as code evolves.
- Scalability: Design tests to be scalable as codebase grows.
- Integration: Integrate unit testing frameworks with development tools and pipelines.
- Best Practices: Follow established best practices and guidelines for effective unit testing.
These aspects are interconnected and contribute to the overall effectiveness of unit testing frameworks. By considering these aspects, developers can improve the quality, reliability, and maintainability of their code.
Modularity
Modularity is a fundamental aspect of implementing unit testing frameworks like JUnit or Pytest for improved code quality. Breaking down code into smaller, testable units allows developers to isolate and test individual components of their codebase, ensuring that each unit functions as expected.
Without modularity, testing complex codebases becomes challenging and error-prone. By breaking down code into smaller units, developers can focus on testing specific functionalities without the need to consider the entire codebase. This approach reduces the risk of introducing bugs and ensures that changes to one unit do not unintentionally affect other parts of the code.
Real-life examples of modularity in unit testing include testing individual methods or classes in isolation. By isolating units, developers can verify their behavior under different inputs and conditions, ensuring that they meet the expected requirements. This approach helps to identify and fix issues early on, preventing them from propagating to other parts of the codebase.
The practical significance of modularity in unit testing is immense. It enables developers to write more effective and maintainable tests, reducing the overall cost of software development and maintenance. By breaking down code into smaller units, developers can quickly identify and fix issues, reducing the risk of defects and improving the overall quality of the software.
Coverage
Coverage, in the context of implementing unit testing frameworks like JUnit or Pytest, refers to the extent to which the tests exercise all possible paths through the code. Achieving high coverage is essential for improving code quality as it helps to identify and eliminate potential issues.
- Test Case Design: Designing test cases that cover all possible scenarios and code paths ensures comprehensive testing.
- Code Coverage Metrics: Using code coverage tools to measure the percentage of code executed by the tests provides visibility into untested areas.
- Branch Coverage: Testing all branches of conditional statements (if-else, switch-case) ensures that all possible outcomes are covered.
- Loop Coverage: Testing loops with different iterations and boundary conditions ensures that loop logic is thoroughly tested.
Achieving high code coverage is not just about increasing the number of tests but about writing tests that effectively exercise all possible code paths. By following best practices for test case design and leveraging code coverage metrics, developers can improve the quality and reliability of their codebase.
Isolation
In unit testing, isolation refers to the practice of testing individual units of code in isolation from other parts of the codebase. This helps to ensure that each unit is functioning correctly and independently, without being affected by external factors or dependencies.
Isolation is a critical component of implementing unit testing frameworks like JUnit or Pytest for improved code quality. By testing units in isolation, developers can identify and fix issues early on, preventing them from propagating to other parts of the codebase. This approach also makes it easier to maintain and refactor code, as changes to one unit are less likely to impact other units.
Real-life examples of isolation in unit testing include testing individual methods or classes in isolation. By isolating units, developers can verify their behavior under different inputs and conditions, ensuring that they meet the expected requirements. This approach helps to identify and fix issues early on, preventing them from propagating to other parts of the codebase.
The practical significance of isolation in unit testing is immense. It enables developers to write more effective and maintainable tests, reducing the overall cost of software development and maintenance. By testing units in isolation, developers can quickly identify and fix issues, reducing the risk of defects and improving the overall quality of the software.
Automation
In the context of implementing unit testing frameworks like JUnit or Pytest for improved code quality, automation plays a crucial role in reducing manual effort and improving efficiency. Automation involves using tools and techniques to execute tests automatically, eliminating the need for manual intervention.
Automation is a critical component of unit testing frameworks as it enables developers to run a large number of tests quickly and consistently. This is especially beneficial for large and complex codebases, where manual testing would be time-consuming and error-prone. Automation also frees up developers to focus on other tasks, such as design and development, rather than spending excessive time on repetitive testing tasks.
Real-life examples of automation in unit testing include using tools such as Maven Surefire or Gradle TestKit to automatically execute tests as part of the build process. These tools allow developers to define test cases and configure test execution parameters, ensuring that tests are run consistently and efficiently.
The practical significance of automation in unit testing is immense. It enables developers to write more effective and maintainable tests, reducing the overall cost of software development and maintenance. By automating tests, developers can quickly identify and fix issues, reducing the risk of defects and improving the overall quality of the software.
In summary, automation is a key aspect of implementing unit testing frameworks for improved code quality. It reduces manual effort, improves efficiency, and enables developers to focus on more complex and creative tasks. By embracing automation, developers can significantly enhance the quality and reliability of their codebase.
Feedback
Providing immediate feedback on test results is critical to enable developers to identify and fix issues early on in the development process, ultimately improving code quality. Here are four key aspects of providing immediate feedback:
- Test Results Display: Displaying test results in a clear and concise format, such as pass/fail indications and error messages, allows developers to quickly assess the outcome of their tests.
- Real-Time Updates: Providing real-time updates on test results as they are executed enables developers to identify issues as they occur, reducing the time to resolution.
- Continuous Integration: Integrating unit tests into continuous integration (CI) pipelines provides developers with immediate feedback on the impact of code changes, ensuring that issues are identified and addressed before merging code.
- Automated Reporting: Generating automated test reports that summarize test results and highlight any failures allows developers to quickly identify areas that need attention and prioritize their efforts.
Immediate feedback on test results empowers developers to take ownership of the quality of their code, leading to more reliable and maintainable software. By incorporating these aspects into unit testing frameworks like JUnit or Pytest, teams can significantly enhance the efficiency and effectiveness of their testing processes.
Maintainability
In the context of implementing unit testing frameworks like JUnit or Pytest, maintainability plays a pivotal role in ensuring that tests remain effective and efficient as the codebase evolves. Maintaining testable code requires proactive measures to prevent tests from becoming brittle and difficult to manage.
- Test Refactoring: As code changes, it is essential to refactor tests alongside the code to ensure they continue to reflect the current behavior of the system. This ensures that tests remain up-to-date and accurate.
- Test Automation: Automating tests reduces the burden of manual maintenance and allows for faster and more efficient execution. Automated tests can be easily triggered as part of the build process, providing quick feedback on code changes.
- Test Organization: Well-organized tests are easier to maintain and debug. Grouping tests logically, using descriptive naming conventions, and employing a consistent structure contributes to maintainability.
- Test Independence: Writing tests that are independent of each other minimizes the impact of code changes on other tests. This promotes isolated testing and reduces the likelihood of cascading test failures.
By adhering to these principles of maintainability, developers can ensure that their unit tests remain effective and efficient over time. This, in turn, contributes to the overall quality and reliability of the codebase, reducing the cost of maintenance and improving the confidence in the software’s functionality.
Scalability
In the realm of “implementing unit testing frameworks like JUnit or Pytest for improved code quality,” scalability plays a crucial role in ensuring that tests remain effective and efficient as the codebase expands. Designing scalable tests involves considering factors such as:
- Test Isolation: Isolating tests from each other minimizes the impact of code changes and prevents cascading test failures, ensuring scalability as the codebase grows.
- Automated Test Execution: Automating test execution reduces the time and effort required to run tests, enabling scalability by allowing for frequent and comprehensive testing even as the codebase expands.
- Parallelized Testing: Parallelizing tests by running them concurrently on multiple threads or machines significantly reduces execution time, enhancing scalability for large codebases.
- Modular Test Design: Decomposing tests into smaller, manageable modules promotes scalability by allowing for independent development, maintenance, and execution of tests.
By incorporating these scalability considerations into unit testing frameworks, developers can ensure that their tests remain effective and efficient even as the codebase grows in size and complexity, ultimately contributing to improved code quality and reduced maintenance costs.
Integration
Integrating unit testing frameworks with development tools and pipelines is a critical aspect of implementing unit testing frameworks like JUnit or Pytest for improved code quality. This integration enables seamless execution of unit tests within the development workflow, enhancing efficiency and ensuring timely feedback on code changes.
Real-life examples of such integration include using Maven Surefire or Gradle TestKit to execute JUnit tests as part of the build process. These tools allow developers to define test cases and configure test execution parameters, ensuring that tests are run consistently and efficiently. Additionally, integrating unit tests with continuous integration (CI) pipelines allows for automated testing as part of the code review and merging process, providing developers with immediate feedback on the impact of code changes.
The practical significance of integrating unit testing frameworks with development tools and pipelines lies in its ability to streamline the testing process and improve code quality. By automating test execution and providing timely feedback, developers can quickly identify and fix issues, reducing the risk of defects and improving the overall reliability of the software. This integration also enables developers to focus on more complex and creative tasks, rather than spending excessive time on repetitive testing tasks.
In summary, integrating unit testing frameworks with development tools and pipelines is a crucial step in implementing effective unit testing practices. This integration streamlines the testing process, improves code quality, and enables developers to focus on more complex and creative tasks.
Best Practices
In the context of implementing unit testing frameworks like JUnit or Pytest for improved code quality, adhering to established best practices and guidelines is of paramount importance. Best practices provide a structured approach to unit testing, ensuring that tests are effective, efficient, and maintainable.
One key aspect of best practices is the use of well-defined naming conventions for test methods and classes. This enhances readability and understanding, making it easier to identify the purpose and scope of each test. Additionally, following guidelines for test organization and structure promotes maintainability, allowing for easier updates and modifications as the codebase evolves.
Furthermore, best practices emphasize the importance of writing atomic and isolated tests. Atomic tests focus on testing a single unit of functionality, while isolated tests ensure that the behavior of one unit is not affected by other parts of the codebase. This approach promotes modularity and reduces the risk of cascading failures.
By following established best practices, developers can ensure that their unit tests are effective in identifying defects, efficient in execution, and maintainable over time. This contributes directly to improved code quality, reduced maintenance costs, and increased confidence in the reliability of the software.
Implementing Unit Testing Frameworks for Improved Code Quality FAQs
This section provides answers to frequently asked questions about implementing unit testing frameworks like JUnit or Pytest. These FAQs address common concerns and misconceptions, clarifying key aspects of unit testing for improved code quality.
Question 1: What are the benefits of implementing unit testing frameworks?
Unit testing frameworks offer numerous benefits, including early detection of defects, improved code quality, reduced maintenance costs, increased development efficiency, and enhanced confidence in the software’s reliability.
Question 2: Which unit testing frameworks are widely used?
JUnit is a popular unit testing framework for Java, while Pytest is commonly used for Python. Both frameworks provide a comprehensive set of features to facilitate effective unit testing.
Question 3: How can I ensure that my unit tests are effective?
To write effective unit tests, focus on testing individual units of functionality in isolation, using atomic and isolated tests. Additionally, follow established best practices for test naming, organization, and structure.
Question 4: How do I integrate unit testing frameworks with my development workflow?
Unit testing frameworks can be integrated with development tools and pipelines, such as Maven Surefire or Gradle TestKit for JUnit, and pytest-cov for Pytest. This integration enables automated test execution and timely feedback on code changes.
Question 5: What is the importance of test coverage?
Test coverage measures the extent to which the tests exercise different paths through the code. Achieving high test coverage helps ensure that all aspects of the code are thoroughly tested, reducing the risk of defects.
Question 6: How can I maintain the quality of my unit tests over time?
To maintain the quality of unit tests, refactor tests alongside code changes, automate test execution, organize tests logically, and ensure test independence. These practices promote maintainability and reduce the burden of test maintenance.
In summary, implementing unit testing frameworks like JUnit or Pytest is crucial for improving code quality. By addressing common questions and misconceptions, these FAQs provide a solid foundation for understanding the benefits, best practices, and integration of unit testing frameworks. These insights contribute to a comprehensive approach to unit testing, enhancing the reliability and maintainability of software applications.
Moving forward, the next section will delve into advanced unit testing techniques, exploring strategies for testing complex scenarios, mocking dependencies, and leveraging code coverage analysis tools.
Tips for Implementing Unit Testing Frameworks for Improved Code Quality
This section provides actionable tips to guide you in effectively implementing unit testing frameworks like JUnit or Pytest for improved code quality. Follow these tips and best practices to enhance the reliability and maintainability of your software applications.
Tip 1: Define Clear and Testable Requirements: Establish well-defined requirements for each unit to ensure that tests accurately reflect the intended functionality.
Tip 2: Isolate Unit Tests: Test units in isolation to minimize dependencies and prevent cascading failures, promoting modular and maintainable tests.
Tip 3: Use Meaningful Test Names: Assign descriptive names to test methods that clearly indicate their purpose, enhancing readability and comprehension.
Tip 4: Leverage Mocking and Stubbing: Utilize mocking and stubbing techniques to create controlled environments for testing, isolating units from external dependencies.
Tip 5: Employ Code Coverage Analysis: Measure test coverage to identify untested areas of the codebase, ensuring comprehensive testing and reducing the risk of defects.
Tip 6: Automate Test Execution: Integrate unit tests into your development pipeline to automate test execution, providing timely feedback on code changes.
Tip 7: Refactor Tests Regularly: Maintain and refactor unit tests alongside code changes to ensure their accuracy and effectiveness over time.
Tip 8: Follow Best Practices and Guidelines: Adhere to established best practices and guidelines for unit testing, such as using standardized naming conventions and organizing tests logically.
By implementing these tips, you can establish a robust unit testing framework that significantly improves code quality, reduces maintenance costs, and enhances confidence in the reliability of your software.
In the concluding section, we will explore advanced unit testing techniques, providing further insights into effective testing strategies and tools.
Conclusion
Implementing unit testing frameworks like JUnit or Pytest is a crucial practice for improving code quality, ensuring software reliability and maintainability. This article explored key aspects of implementing unit testing frameworks, including modularity, coverage, isolation, automation, feedback, maintainability, scalability, integration, and best practices. By following these principles and utilizing effective techniques and tools, developers can establish a robust unit testing framework that significantly enhances the quality of their codebase.
Two main points from the article are the importance of isolating unit tests to prevent cascading failures and the use of code coverage analysis to ensure comprehensive testing. These points are interconnected, as isolating tests promotes modularity and maintainability, while code coverage analysis helps identify areas that may not be adequately tested. By addressing both aspects, developers can create a unit testing framework that effectively identifies and addresses potential issues.
In summary, implementing unit testing frameworks is an essential aspect of modern software development. By embracing the principles, techniques, and tools discussed in this article, developers can improve the quality, reliability, and maintainability of their codebase, ultimately leading to more robust and dependable software applications.
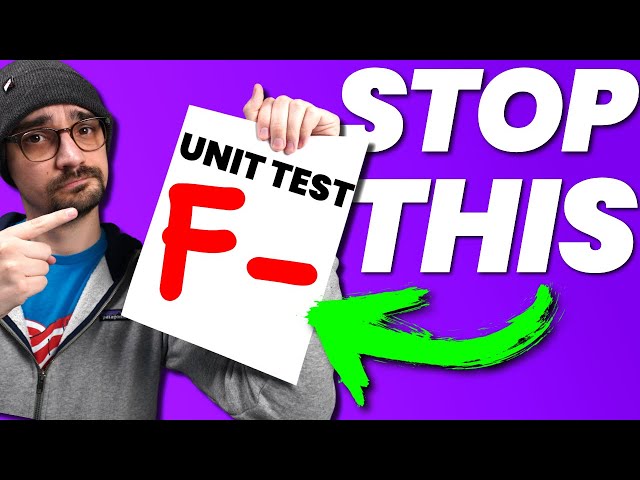