Debugging Tips and Tricks for Troubleshooting Common Programming Errors
Debugging is the process of analyzing a program to find and correct errors. Tips and tricks can help make debugging more efficient and effective.
Debugging is an essential part of software development. It helps ensure that programs are correct and reliable. One of the most important developments in debugging was the introduction of the debugger.
This article will discuss some common debugging tips and tricks. It will also provide some advice on how to use a debugger.
Debugging Tips and Tricks for Troubleshooting Common Programming Errors
Debugging is an essential part of software development. It helps ensure that programs are correct and reliable. There are many different debugging tips and tricks that can help you troubleshoot common programming errors.
- Use a debugger
- Set breakpoints
- Examine the call stack
- Use logging
- Test your code regularly
- Use version control
- Ask for help
- Learn from your mistakes
- Be patient
- Don’t give up
These are just a few of the many debugging tips and tricks that can help you troubleshoot common programming errors. By following these tips, you can save time and frustration, and write better code.
Use a debugger
A debugger is a tool that helps you debug your code. It allows you to step through your code line by line, examine the values of variables, and set breakpoints.
Using a debugger is one of the most effective ways to troubleshoot common programming errors. It can help you quickly identify the source of an error and fix it.
There are many different debuggers available, both commercial and open source. Some popular debuggers include GDB, LLDB, and Visual Studio Debugger.
If you are new to debugging, I recommend starting with a simple debugger like GDB or LLDB. Once you have become more familiar with debugging, you may want to try a more advanced debugger like Visual Studio Debugger.
Using a debugger can save you a lot of time and frustration when troubleshooting programming errors. It is a valuable tool that every programmer should learn to use.
Set breakpoints
Setting breakpoints is a debugging technique that allows you to pause the execution of your program at a specific point. This can be useful for examining the values of variables, inspecting the call stack, and identifying the source of an error.
-
Location
You can set breakpoints at specific lines of code, or at specific addresses in memory.
-
Conditional
You can set conditional breakpoints that only trigger when certain conditions are met.
-
Temporary
You can set temporary breakpoints that are only active for a single debugging session.
-
Persistent
You can set persistent breakpoints that are saved between debugging sessions.
Setting breakpoints is a powerful debugging technique that can help you quickly identify and fix errors in your code. It is a valuable tool that every programmer should learn to use.
Examine the call stack
Examining the call stack is a debugging technique that allows you to see the sequence of function calls that led to the current state of your program. This can be useful for identifying the source of an error, or for understanding how your program is executing.
-
Function Calls
The call stack shows the sequence of function calls that have been made, starting with the main function and ending with the current function.
-
Arguments and Return Values
The call stack also shows the arguments that were passed to each function, and the return values that were returned.
-
Local Variables
The call stack can also show the local variables that are defined in each function.
-
Call Stack Depth
The call stack depth is the number of functions that have been called before the current function. This can be useful for identifying recursive functions or infinite loops.
Examining the call stack is a powerful debugging technique that can help you quickly identify and fix errors in your code. It is a valuable tool that every programmer should learn to use.
Use logging
Logging is an essential debugging technique that allows you to record the execution of your program and identify any errors or issues. It is a valuable tool that can help you quickly identify and fix problems in your code.
-
Log Levels
Logging allows you to specify the level of detail that is logged. This can be useful for filtering out less important information and focusing on the most critical errors.
-
Log Files
Logs can be written to files, which can be helpful for long-running programs or for storing logs for later analysis.
-
Log Parsers
There are a number of tools available that can help you parse and analyze log files. This can be useful for identifying patterns and trends in your logs.
-
Log Aggregation
Log aggregation tools can help you collect and centralize logs from multiple sources. This can be useful for managing and analyzing logs from large distributed systems.
Logging is a powerful debugging technique that can help you quickly identify and fix errors in your code. It is a valuable tool that every programmer should learn to use.
Test your code regularly
Testing your code regularly is one of the most important debugging tips and tricks. By testing your code regularly, you can identify and fix errors early on, before they cause major problems.
There are many different ways to test your code. You can write unit tests, integration tests, and end-to-end tests. You can also use a testing framework like JUnit or NUnit to help you write and run your tests.
No matter how you choose to test your code, the important thing is to test it regularly. By testing your code regularly, you can help ensure that your code is correct and reliable.
Use version control
Using version control is an essential part of any software development process. It allows you to track changes to your code over time, and to easily revert to previous versions if necessary.
-
Collaboration
Version control allows multiple developers to work on the same project simultaneously, without overwriting each other’s changes.
-
History
Version control keeps a history of all changes to your code. This can be useful for debugging purposes, as it allows you to see what changes were made and when.
-
Rollback
If you make a mistake, you can easily roll back to a previous version of your code. This can save you a lot of time and frustration.
-
Branching
Version control allows you to create branches of your code. This can be useful for experimenting with new features or bug fixes without affecting the main branch of your code.
Using version control is a valuable debugging tip that can help you save time and frustration. It is a good practice to use version control for all of your software development projects.
Ask for help
Asking for help is an essential part of the debugging process. No matter how experienced you are, there will always be times when you get stuck and need help from someone else. There are many different ways to ask for help, such as posting a question on a forum, asking a colleague for help, or hiring a professional debugger.
There are many benefits to asking for help. First, it can save you a lot of time and frustration. Second, it can help you learn new things and improve your debugging skills. Third, it can help you build relationships with other developers and create a sense of community.
If you are struggling to debug a problem, don’t be afraid to ask for help. There are many people who are willing to help you, and they can save you a lot of time and frustration.
Learn from your mistakes
Learning from your mistakes is an essential part of the debugging process. By taking the time to analyze your mistakes, you can improve your debugging skills and avoid making the same mistakes in the future.
-
Identify the root cause
The first step to learning from your mistakes is to identify the root cause of the error. This can be challenging, but it is essential for preventing the same mistake from happening again.
-
Understand the implications
Once you have identified the root cause of the error, you need to understand the implications of the error. This includes understanding how the error affected the program and what could have been done to prevent it.
-
Develop a solution
Once you understand the implications of the error, you need to develop a solution to prevent it from happening again. This may involve changing your code, adding new tests, or improving your debugging skills.
-
Test your solution
Once you have developed a solution, you need to test it to ensure that it works. This may involve running your code through a series of tests or using a debugger to step through your code.
Learning from your mistakes is an essential part of the debugging process. By taking the time to analyze your mistakes, you can improve your debugging skills and avoid making the same mistakes in the future.
Be patient
Debugging can be a frustrating and time-consuming process. It is important to be patient and to not give up when you encounter errors. By taking the time to carefully examine your code and to use the debugging tips and tricks described in this article, you can eventually find and fix any errors in your code.
-
Take breaks
If you are stuck on a debugging problem, it can be helpful to take a break and come back to it later. This can help you to clear your head and to see the problem from a fresh perspective.
-
Don’t be afraid to ask for help
If you are struggling to debug a problem, don’t be afraid to ask for help from a colleague, friend, or online forum. There are many people who are willing to help others to debug their code.
-
Don’t give up
It is important to be persistent when debugging. Don’t give up if you can’t find the error right away. Keep working at it and you will eventually find the solution.
By following these tips, you can become a more patient and effective debugger. This will help you to save time and frustration, and to write better code.
Don’t give up
Debugging is a crucial aspect of software development, and perseverance plays a pivotal role in this process. “Don’t give up” is a key principle that guides effective debugging, encompassing several facets that contribute to successful troubleshooting.
-
Persistence
Debugging often involves encountering obstacles and setbacks. Persistence is essential to overcome these challenges, ensuring that the debugging process is carried out thoroughly and efficiently. -
Break down the problem
Complex debugging issues can be overwhelming. Breaking them down into smaller, manageable chunks makes them less daunting and easier to resolve. -
Take breaks
Continuous debugging can lead to mental fatigue. Taking breaks allows the mind to refresh, providing a renewed perspective when returning to the task. -
Seek support
Collaboration and seeking assistance from peers, online forums, or documentation can provide valuable insights and alternative approaches to debugging problems.
Embracing the principle of “Don’t give up” empowers programmers to approach debugging with determination and resilience. By adopting these facets, they can enhance their debugging skills, minimize frustrations, and ultimately deliver high-quality software products.
Frequently Asked Questions About Debugging Tips and Tricks for Troubleshooting Common Programming Errors
This FAQ section addresses common questions and clarifies key aspects of debugging tips and tricks for troubleshooting common programming errors.
Question 1: What are some essential debugging tips for beginners?
Answer: Utilizing a debugger, setting breakpoints, examining the call stack, and employing logging techniques are fundamental debugging practices.
Question 2: How can I improve my debugging skills?
Answer: Regularly testing your code, utilizing version control, and learning from your mistakes are effective ways to enhance your debugging abilities.
Question 3: What are some common programming errors that I should be aware of?
Answer: Syntax errors, runtime errors, and logical errors are prevalent types of programming errors to watch out for.
Question 4: How can I troubleshoot errors in a large codebase?
Answer: Employing debugging tools like debuggers and profilers, utilizing logging and error handling mechanisms, and adopting a divide-and-conquer approach can aid in troubleshooting large codebases.
Question 5: What are some resources for learning more about debugging?
Answer: Online documentation, tutorials, books, and forums provide valuable resources for expanding your debugging knowledge.
Question 6: How can I prevent debugging from becoming a time-consuming task?
Answer: Adopting good coding practices, leveraging automated testing, and seeking assistance when needed can help minimize debugging time.
These FAQs provide a foundation for understanding common debugging tips and tricks. In the next section, we will delve deeper into advanced debugging techniques for resolving complex programming errors.
Debugging Tips and Tricks
This section provides practical and actionable tips to assist you in effectively troubleshooting common programming errors.
Tip 1: Utilize a Debugger
Employ debugging tools like GDB or LLDB to step through your code line by line, examining variable values and identifying errors.
Tip 2: Set Strategic Breakpoints
Place breakpoints at specific code locations or conditions to pause execution and inspect the program’s state.
Tip 3: Examine the Call Stack
Analyze the sequence of function calls to trace the path of execution and identify the source of errors.
Tip 4: Leverage Logging Effectively
Implement logging statements to record program execution details, enabling you to review and pinpoint issues.
Tip 5: Practice Regular Code Testing
Conduct thorough testing to proactively detect and resolve errors before they manifest in production.
Tip 6: Utilize Version Control Systems
Employ version control tools like Git to track code changes, allowing you to revert to previous versions if errors arise.
Tip 7: Seek Assistance When Needed
Collaborate with peers, consult online forums, or engage professional debugging services when encountering complex issues.
Tip 8: Learn from Debugging Experiences
Analyze errors and their resolutions to enhance your debugging skills and prevent future occurrences.
These tips empower you to approach debugging systematically, reducing time spent on troubleshooting and improving the quality of your software products.
In the next section, we will explore advanced debugging techniques to tackle intricate programming challenges.
Conclusion
Effective debugging is vital for developing high-quality software. This article provided comprehensive insights into debugging tips and tricks, empowering programmers to troubleshoot common programming errors efficiently.
Key takeaways include the importance of utilizing debuggers, setting strategic breakpoints, and leveraging logging to pinpoint errors. Regular code testing and version control practices enhance debugging effectiveness. Seeking assistance and learning from debugging experiences foster continuous improvement.
By applying these principles, programmers can transform debugging from a daunting task into a proactive and productive aspect of software development, ensuring the reliability and performance of their software products.
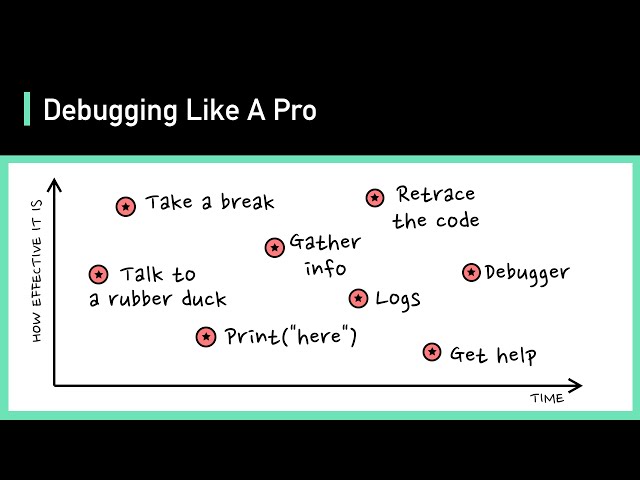