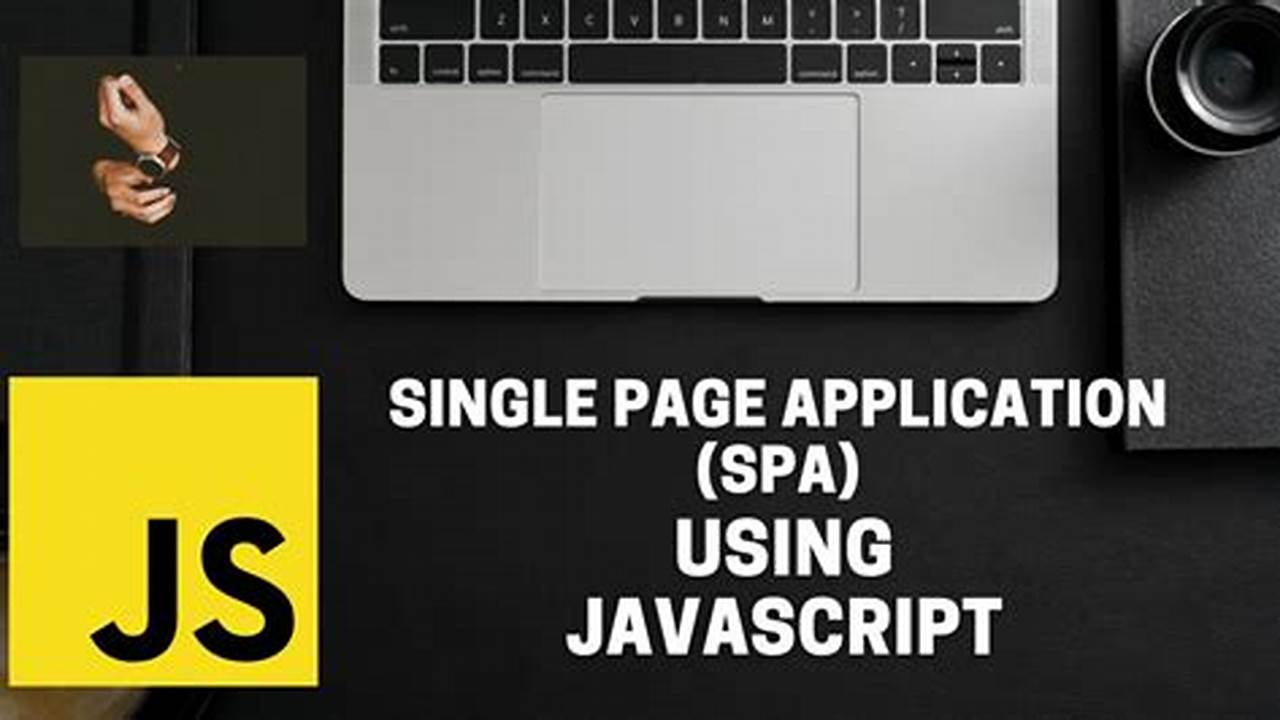
Creating a Single Page Application (SPA) with React JS and Redux for Enhanced User Experience
Modern web applications demand seamless and responsive user experiences. A single-page application (SPA) built with React JS, a popular JavaScript framework, and Redux, a state management system, allows developers to create dynamic and interactive web experiences.
SPAs load all necessary resources on a single page, eliminating the need for page refreshes, and providing a smooth and uninterrupted user journey. React JS’s component-based architecture and Redux’s centralized state management capabilities enable efficient and scalable application development. This approach enhances user engagement, provides a mobile-friendly experience, and optimizes performance.
This article delves into the intricacies of creating SPAs using React JS and Redux, exploring best practices, performance optimizations, and advanced techniques to unlock the full potential of this powerful development stack.
Creating a Single Page Application (SPA) with React JS and Redux for Improved User Experience
Creating a SPA with React JS and Redux involves several key aspects that contribute to an enhanced user experience. These aspects encompass various dimensions, including architecture, performance, and state management.
- Component-based Architecture
- Virtual DOM
- State Management
- Data Fetching
- Routing
- Performance Optimization
- Code Splitting
- Error Handling
- Testing
- Deployment
Component-based architecture allows for modular and reusable code, while the Virtual DOM ensures efficient updates. State management with Redux provides centralized control and predictability. Data fetching and routing enable seamless navigation and data retrieval. Performance optimization techniques, such as code splitting, enhance load times and responsiveness. Error handling ensures graceful degradation and user-friendliness. Testing guarantees code stability and reliability. Deployment considerations ensure smooth transitions to production environments.
Component-based Architecture
Component-based architecture is a fundamental aspect of creating single-page applications (SPAs) with React JS and Redux. It involves breaking down the user interface (UI) into reusable and independent components, each responsible for a specific functionality or presentation.
This approach offers several advantages. Firstly, it promotes code reusability, reducing development time and maintenance effort. Secondly, it enhances modularity, allowing developers to work on different components independently, improving collaboration and code maintainability. Thirdly, the component-based architecture facilitates the creation of complex UIs by assembling smaller, well-defined components.
In the context of React JS, components are defined as reusable UI elements that can be composed together to create larger, more complex UIs. React’s Virtual DOM ensures efficient updates, minimizing the impact of changes on the UI. Redux, as a state management tool, provides centralized control over the application’s state, enabling predictable and consistent behavior. Together, these technologies empower developers to build robust and scalable SPAs with improved user experience.
Virtual DOM
Within the context of creating single-page applications (SPAs) with React JS and Redux, the Virtual DOM plays a pivotal role in enhancing user experience. It serves as an in-memory representation of the real DOM, enabling efficient updates and optimizations.
- Lightweight RepresentationThe Virtual DOM is a lightweight data structure that mirrors the real DOM, representing only the necessary information for UI rendering. This reduces the overhead associated with manipulating the actual DOM, resulting in faster updates and improved performance.
- Efficient Diffing AlgorithmReact employs a different algorithm to compare the previous Virtual DOM with the updated one, identifying only the minimal changes required to the real DOM. This optimization minimizes the number of DOM manipulations, leading to smoother and more responsive UI interactions.
- Batching UpdatesReact batches multiple state updates into a single render pass, preventing unnecessary re-renders and improving overall performance. This is particularly beneficial in scenarios with frequent state changes, such as when handling user inputs or real-time data updates.
- Server-side RenderingThe Virtual DOM enables server-side rendering, allowing SPAs to be initially rendered on the server. This technique improves the perceived performance by delivering a fully rendered page to the client, reducing the initial loading time, and providing a better user experience.
In summary, the Virtual DOM is a key aspect of creating SPAs with React JS and Redux due to its lightweight representation, efficient diffing algorithm, batching capabilities, and support for server-side rendering. These features combined contribute to a more responsive, performant, and user-friendly application experience.
State Management
In the context of creating single-page applications (SPAs) with React JS and Redux, state management plays a pivotal role in enhancing user experience. State refers to the data that describes the current of the application, such as user input, server responses, and UI settings. Effective state management ensures that the application’s state is consistent, predictable, and accessible throughout the application.
Redux is a popular state management tool for React applications. It provides a centralized store for the application state, enabling developers to manage and update the state in a structured and controlled manner. Redux follows a unidirectional data flow, where actions are dispatched to the store, which then predictably updates the state. This approach simplifies state management, reduces the risk of inconsistencies, and enhances application maintainability.
Real-life examples of state management in SPAs with React JS and Redux include managing user authentication, shopping cart items, and form data. By centralizing the state in a Redux store, developers can easily track changes, handle asynchronous operations, and ensure data consistency across the application. This leads to a more seamless and responsive user experience, as the application can react to user actions and data updates in a predictable and timely manner.
Understanding the connection between state management and creating SPAs with React JS and Redux is crucial for building robust and maintainable applications. It enables developers to manage the application’s state effectively, ensuring data consistency, predictable behavior, and improved user experience. By leveraging the capabilities of Redux, developers can create SPAs that are responsive, scalable, and capable of handling complex state management scenarios.
Data Fetching
Data fetching is a crucial aspect of creating single-page applications (SPAs) with React JS and Redux, enabling the retrieval of data from various sources to populate the application’s UI and drive its functionality.
- Data SourcesData fetching involves retrieving data from different sources, such as REST APIs, databases, or local storage. This data can include user information, product catalogs, or real-time updates, which are essential for building dynamic and interactive SPAs.
- Asynchronous RequestsData fetching typically involves asynchronous requests, where the application can continue executing while waiting for the data to be retrieved. This non-blocking approach prevents the UI from freezing and ensures a responsive user experience, even when dealing with large datasets or slow network connections.
- Data NormalizationData fetching often requires normalization, which involves converting data from different sources into a consistent format. This ensures that the data can be easily integrated into the application’s state management system and used across various components.
- Error HandlingRobust error handling is essential for data fetching, as network issues or server errors can occur. Proper error handling prevents the application from crashing and provides meaningful feedback to users, enhancing the overall user experience.
Effective data fetching practices are fundamental for building SPAs with React JS and Redux. By implementing efficient asynchronous data retrieval, ensuring consistent data formatting, and handling errors gracefully, developers can create applications that are responsive, reliable, and capable of handling complex data requirements.
Routing
Routing plays a vital role in creating single-page applications (SPAs) with React JS and Redux. It enables the application to handle navigation between different views or pages without the need for full-page refreshes, providing a seamless and responsive user experience.
React Router is a popular routing library for React applications. It provides a declarative approach to defining application routes, making it easy to specify the mapping between URLs and components. This declarative approach simplifies the development and maintenance of complex navigation structures, as developers can define routes straightforwardly and intuitively.
An essential aspect of routing in SPAs is the use of client-side routing. Unlike traditional server-side routing, client-side routing handles navigation within the application without involving the server. This approach significantly improves performance and responsiveness, as there is no need to wait for the server to render a new page. React Router’s client-side routing capabilities enable SPAs to provide a fast and fluid navigation experience.
In summary, routing is a critical component of creating SPAs with React JS and Redux. It allows developers to define navigation routes declaratively, leveraging React Router’s capabilities for client-side routing. By embracing client-side routing, SPAs can achieve improved performance, responsiveness, and a seamless user experience.
Performance Optimization
Performance optimization is a crucial aspect of creating single-page applications (SPAs) with React JS and Redux, as it directly impacts the user experience. By optimizing performance, developers can ensure that their applications are responsive, smooth, and efficient, leading to increased user satisfaction and engagement.
- Code SplittingCode splitting involves dividing the application code into smaller, manageable chunks that can be loaded on demand. This reduces the initial load time and improves the overall performance of the application, especially on slower networks.
- Lazy LoadingLazy loading is a technique where components or modules are only loaded when they are needed. This approach reduces the initial load time and memory footprint of the application, improving performance and optimizing resource utilization.
- CachingCaching involves storing frequently used data or resources in memory for faster access. This can significantly improve the performance of the application by reducing the need to fetch data from the server or perform expensive calculations.
- Profiling and Performance MonitoringProfiling and performance monitoring tools help identify performance bottlenecks and pinpoint areas for optimization. By analyzing performance metrics and identifying performance issues, developers can make informed decisions to improve the efficiency of their applications.
Performance optimization is an ongoing process that involves a combination of techniques and best practices. By implementing these optimizations, developers can create SPAs that are performant, responsive, and provide an enhanced user experience.
Code Splitting
Code splitting is a critical performance optimization technique used in creating single-page applications (SPAs) with React JS and Redux. It involves dividing the application code into smaller, manageable chunks, which are then loaded on demand as needed. This approach reduces the initial load time of the application, leading to a faster and more responsive user experience.
In the context of SPAs, code splitting plays a particularly important role due to the single-page nature of these applications. Unlike traditional multi-page applications, SPAs load all the necessary resources on a single page, which can result in long load times if the codebase is large. Code splitting addresses this issue by breaking down the application into smaller, more manageable modules that can be loaded asynchronously as the user navigates through the application.
A real-life example of code splitting in SPAs is the use of lazy loading. With lazy loading, individual components or modules of the application are only loaded when they are needed. This technique further reduces the initial load time and improves the overall performance of the application, especially on slower networks or devices.
Understanding the connection between code splitting and creating SPAs with React JS and Redux is crucial for developers who want to build high-performing and user-friendly applications. By implementing code-splitting techniques, developers can reduce load times, improve responsiveness, and enhance the overall user experience of their SPAs.
Error Handling
Error handling plays a critical role in creating single-page applications (SPAs) with React JS and Redux, as it directly impacts the user experience, application stability, and overall reliability. Effective error-handling strategies ensure that errors are handled gracefully, providing meaningful feedback to users and preventing the application from crashing.
In SPAs, error handling is crucial due to the potential for various errors to occur during the application’s lifecycle. These errors can range from network issues and server errors to user input validation errors. Robust error-handling mechanisms allow developers to anticipate and handle these errors proactively, preventing them from disrupting the user experience.
A real-life example of error handling in SPAs is the use of error boundaries. Error boundaries are React components that catch errors within their child components, preventing the entire application from crashing. When an error occurs, the error boundary displays a fallback UI or error message, allowing the user to continue using the application while the error is being addressed.
Understanding the connection between error handling and creating SPAs with React JS and Redux is essential for building reliable and user-friendly applications. By implementing effective error-handling strategies, developers can improve the overall quality and stability of their SPAs, ensuring that users encounter minimal disruptions or frustrations while using the application.
Testing
In the context of creating single-page applications (SPAs) with React JS and Redux for improved user experience, testing plays a critical role in ensuring the quality, stability, and reliability of the application. Testing involves evaluating the application’s behavior under various conditions and scenarios to identify and fix potential issues before they reach production.
Testing is a crucial component of the development process for SPAs due to the dynamic and interactive nature of these applications. SPAs rely heavily on client-side code and asynchronous operations, which can introduce potential sources of errors if not properly tested. By implementing comprehensive testing strategies, developers can proactively identify and address these issues, resulting in a more robust and user-friendly application.
Real-life examples of testing within SPAs include unit testing individual components, integration testing to verify interactions between components, and end-to-end testing to simulate real-user scenarios. Unit testing allows developers to isolate and test individual components, ensuring that they function as intended. Integration testing verifies the correct behavior of components when working together, while end-to-end testing provides a comprehensive evaluation of the application’s overall functionality and user experience.
Understanding the connection between testing and creating SPAs with React JS and Redux is essential for building high-quality and reliable applications. By embracing a rigorous testing approach, developers can proactively identify and fix issues, prevent regressions, and improve the overall user experience. This leads to increased confidence in the application’s stability, reduced maintenance costs, and enhanced user satisfaction.
Deployment
Deployment, in the context of creating single-page applications (SPAs) with React JS and Redux, refers to the process of making the application available to users in a production environment. It involves packaging the application code, configuring servers, and implementing measures to ensure the application’s stability and performance.
Deployment plays a critical role in the overall user experience of SPAs. A well-deployed application is accessible, reliable, and performant, meeting the expectations of end-users. Poor deployment practices, on the other hand, can lead to downtime, errors, and a degraded user experience.
Real-life examples of deployment within SPAs include deploying the application to a cloud hosting provider, such as AWS or Azure, or deploying to a dedicated server. The choice of deployment platform depends on factors such as the size and complexity of the application, traffic volume, and cost considerations.
Understanding the connection between deployment and creating SPAs with React JS and Redux is essential for ensuring the success of the application. By adopting best practices for deployment, developers can deliver a high-quality user experience, minimize downtime, and respond effectively to changes in user demand or application updates.
FAQs on Creating Single-Page Applications (SPAs) with React JS and Redux
This FAQ section provides answers to common questions and clarifies key aspects of creating SPAs with React JS and Redux. It aims to address potential queries and offer insights to enhance the user experience.
Question 1: What are the key benefits of using React JS and Redux for SPAs?
React JS offers component-based architecture, enabling modular and reusable code. Redux provides centralized state management, ensuring predictability and consistency. Together, they enhance performance, simplify maintenance, and improve user experience.
Question 2: How does code splitting improve the performance of SPAs?
Code splitting divides the application code into smaller chunks, which are loaded on demand. This reduces the initial load time and improves responsiveness, especially for large applications or slow network connections.
Question 3: What is the role of Redux in state management for SPAs?
Redux provides a centralized store for the application state, enabling controlled and predictable updates. It follows a unidirectional data flow, simplifying state management and reducing the risk of inconsistencies.
Question 4: How does error handling enhance the user experience in SPAs?
Robust error handling prevents application crashes and provides meaningful feedback to users. It ensures that errors are handled gracefully, maintaining a positive user experience even in the presence of unexpected issues.
Question 5: What is the significance of testing in the development of SPAs?
Testing helps identify and fix potential issues before they reach production, ensuring the stability and quality of the application. It involves unit testing, integration testing, and end-to-end testing to evaluate different aspects of the application’s functionality.
Question 6: How does deployment affect the user experience of SPAs?
Deployment involves making the application accessible to users. Proper deployment ensures reliability, performance, and accessibility. Poor deployment practices can lead to downtime or degraded user experience.
These FAQs provide insights into the key aspects of creating SPAs with React JS and Redux, highlighting the importance of performance optimization, state management, error handling, testing, and deployment in delivering an enhanced user experience.
In the next section, we will explore advanced techniques and best practices for building robust and scalable SPAs with React JS and Redux.
Tips for Creating Single-Page Applications (SPAs) with React JS and Redux for Improved User Experience
This section provides actionable tips to enhance the user experience of SPAs built with React JS and Redux. By implementing these best practices, developers can create high-performing, reliable, and user-friendly applications.
Tip 1: Utilize Code Splitting: Divide the application code into smaller chunks and load them on demand to reduce initial load time and improve responsiveness.
Tip 2: Implement Effective Error Handling: Handle errors gracefully using error boundaries or other mechanisms to prevent application crashes and provide meaningful feedback to users.
Tip 3: Optimize Data Fetching: Employ efficient data fetching techniques, such as lazy loading and caching, to minimize the impact on performance and ensure a smooth user experience.
Tip 4: Leverage Redux for Centralized State Management: Use Redux to manage the application state in a centralized and predictable manner, simplifying state updates and reducing the risk of inconsistencies.
Tip 5: Prioritize Performance Optimization: Implement performance optimization techniques, such as code splitting and lazy loading, to enhance the responsiveness and perceived speed of the application.
Tip 6: Conduct Thorough Testing: Perform comprehensive testing, including unit testing, integration testing, and end-to-end testing, to identify and fix potential issues before they reach production, ensuring application stability.
Tip 7: Employ Best Practices for Deployment: Deploy the application using reliable hosting providers and implement measures for scalability, security, and monitoring to ensure a seamless user experience.
Summary: By incorporating these tips into their development process, developers can create SPAs with React JS and Redux that are performant, reliable, and offer an enhanced user experience. These practices improve the overall quality, stability, and accessibility of the application.
The next section will discuss advanced techniques and best practices for building robust and scalable SPAs with React JS and Redux, further enhancing the user experience and application performance.
Conclusion
This article explored the topic of “creating a single page application (SPA) with React JS and Redux for improved user experience.” SPAs offer several advantages, including enhanced performance, seamless navigation, and a more engaging user experience. React JS and Redux are powerful technologies that enable developers to build robust and scalable SPAs.
Key points discussed in this article include:
- Utilizing React JS’s component-based architecture for modular and reusable code.
- Implementing Redux for centralized state management, ensuring predictability and consistency.
- Employing techniques such as code splitting and lazy loading to optimize performance and enhance responsiveness.
By leveraging these technologies and best practices, developers can create SPAs that deliver an exceptional user experience. SPAs are the future of web development, and React JS and Redux provide the tools necessary to build high-quality applications that meet the demands of modern users.
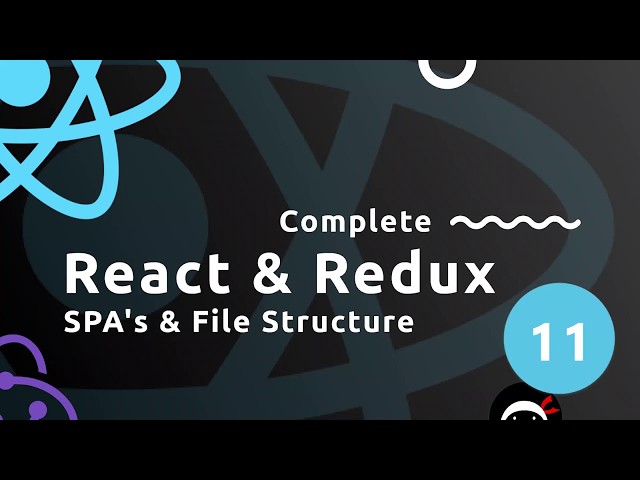