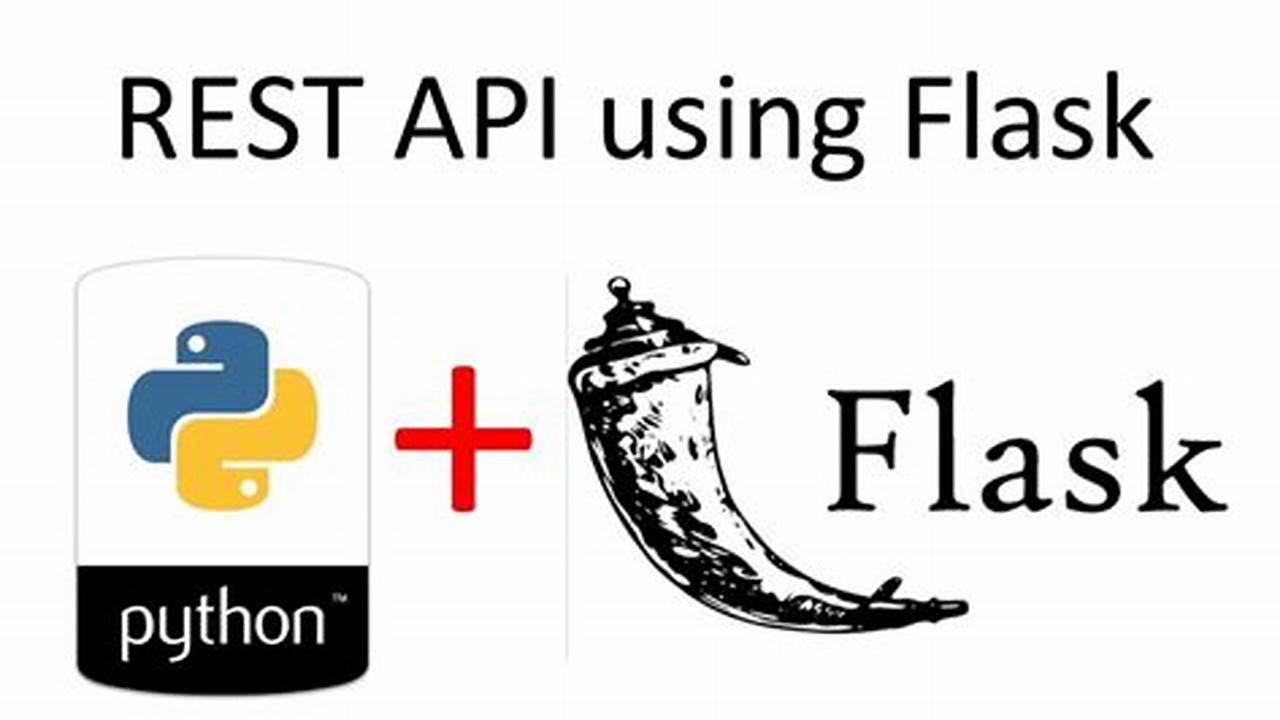
Building RESTful APIs with Python and Flask for Efficient Data Exchange
Building RESTful APIs with Python and Flask is a powerful technique for creating efficient and scalable data exchange solutions. A RESTful API (Representational State Transfer Application Programming Interface) is a set of protocols that define how data is transferred over the internet. By utilizing Python, a versatile programming language, and Flask, a lightweight web framework, developers can craft robust APIs that adhere to RESTful principles. This approach enables seamless data exchange between various applications and services, fostering interoperability and streamlining data management.
This article delves into the significance of building RESTful APIs with Python and Flask, exploring their advantages and tracing their historical evolution. It also provides guidance on implementing this approach in practice, leveraging Python’s extensive libraries and Flask’s user-friendly features. By understanding the concepts and techniques presented herein, developers can harness the power of RESTful APIs to unlock new possibilities for data exchange and application integration.
Building RESTful APIs with Python and Flask for Efficient Data Exchange
Building RESTful APIs with Python and Flask involves several essential aspects that contribute to their efficiency and effectiveness in data exchange. These aspects encompass various dimensions, ranging from the technical foundations to the practical implementation and optimization strategies.
- Architecture: Layered design for scalability and maintainability.
- Data Modeling: Defining data structures and relationships for efficient data representation.
- Routing: Mapping URLs to API endpoints for handling client requests.
- Serialization: Converting data into a format suitable for transmission (e.g., JSON, XML).
- Security: Implementing authentication, authorization, and encryption for data protection.
- Testing: Ensuring API functionality and correctness through automated tests.
- Documentation: Providing clear and comprehensive documentation for API usage.
- Performance Optimization: Employing caching, pagination, and other techniques to enhance API responsiveness.
These aspects are interconnected and play a vital role in shaping the overall quality and efficiency of RESTful APIs built with Python and Flask. By carefully considering and implementing these aspects, developers can create robust and scalable data exchange solutions that meet the demands of modern applications and services.
Architecture
In the context of building RESTful APIs with Python and Flask for efficient data exchange, architecture plays a pivotal role in ensuring scalability and maintainability. A well-structured, layered architecture forms the foundation for robust and extensible APIs that can handle growing data volumes and evolving requirements.
A layered architecture involves organizing the API into distinct layers, each responsible for a specific set of functionality. Common layers include the data access layer, business logic layer, and presentation layer. This separation of concerns promotes code reusability, simplifies maintenance, and facilitates the integration of new features or services.
For example, in a RESTful API built with Python and Flask, the data access layer might handle database interactions, the business logic layer might implement the API’s core functionality, and the presentation layer might format and return data in the appropriate format (e.g., JSON, XML). This layered approach enables developers to make changes to one layer without affecting the others, reducing the risk of errors and improving overall API stability.
Overall, adopting a layered design for architecture is a critical component of building RESTful APIs with Python and Flask for efficient data exchange. It promotes scalability by allowing the API to handle increasing data volumes and complexity, and it enhances maintainability by facilitating code reusability and reducing the impact of future changes.
Data Modeling
In the context of building RESTful APIs with Python and Flask for efficient data exchange, data modeling plays a crucial role in ensuring the efficient representation and manipulation of data. A well-defined data model provides a blueprint for the structure and relationships within the data, enabling the API to process and exchange data effectively.
- Data Structures: Defining the types and formats of data elements, such as integers, strings, booleans, and complex objects.
- Relationships: Establishing connections between data elements, such as one-to-many or many-to-many relationships, to represent real-world entities and their interactions.
- Normalization: Decomposing data into smaller, more manageable tables to reduce redundancy and improve data integrity.
- Data Validation: Implementing rules and constraints to ensure that data conforms to expected formats and business requirements.
By carefully considering and implementing these aspects of data modeling, developers can create RESTful APIs with Python and Flask that are capable of handling complex data structures, representing real-world relationships, and maintaining data integrity. This, in turn, contributes to the overall efficiency and effectiveness of data exchange, enabling applications and services to seamlessly share and process data.
Routing
In the context of building RESTful APIs with Python and Flask for efficient data exchange, routing plays a critical role in ensuring that client requests are directed to the appropriate API endpoints for processing. Routing involves mapping specific URLs to specific API endpoints, which are responsible for handling the corresponding requests and returning the appropriate responses.
Routing is a fundamental component of building RESTful APIs with Python and Flask, as it enables the API to handle a wide range of client requests and provide the desired data or functionality. Without proper routing, clients would not be able to access the API’s resources or perform the desired operations.
Real-life examples of routing in RESTful APIs with Python and Flask include:
- Mapping the URL “/api/users” to an API endpoint that handles user-related requests, such as creating, retrieving, updating, or deleting users.
- Mapping the URL “/api/products” to an API endpoint that handles product-related requests, such as fetching product details, adding products to a shopping cart, or processing orders.
Understanding the connection between routing and building RESTful APIs with Python and Flask for efficient data exchange is crucial for developers who want to create robust and scalable APIs. Proper routing ensures that client requests are handled efficiently and effectively, contributing to the overall performance and user experience of the API.
Serialization
Serialization plays a vital role in building RESTful APIs with Python and Flask for efficient data exchange. It involves converting data into a format that can be easily transmitted over the network, ensuring seamless communication between the API and client applications.
- Data Formats: Serialization supports various data formats, such as JSON (JavaScript Object Notation) and XML (Extensible Markup Language), enabling data exchange in a structured and platform-independent manner.
- Object-to-JSON Conversion: Python provides libraries like `json` to convert Python objects into JSON format, simplifying data transfer and reducing the need for manual formatting.
- Performance Considerations: The choice of serialization format can impact API performance. JSON is generally faster to parse and generate than XML, making it a suitable choice for high-volume data exchange.
- Error Handling: Serialization can introduce errors if data is not properly formatted or if the receiving application cannot parse the data correctly. Implementing robust error handling mechanisms is crucial to ensure data integrity and API reliability.
By understanding and leveraging the aspects of serialization discussed above, developers can build RESTful APIs with Python and Flask that efficiently exchange data in a variety of formats. This contributes to the overall performance, interoperability, and reliability of the API, enabling seamless data exchange between different applications and services.
Security
In the context of building RESTful APIs with Python and Flask for efficient data exchange, security is paramount. Implementing robust security measures ensures that data is protected from unauthorized access, modification, or disclosure, maintaining the integrity and confidentiality of sensitive information.
- Authentication: Verifying the identity of users or clients accessing the API, typically through mechanisms like passwords, tokens, or certificates.
- Authorization: Controlling the access rights of authenticated users to specific resources or operations within the API, ensuring that users can only perform actions they are authorized to.
- Encryption: Encrypting data at rest and in transit, safeguarding it from unauthorized interception or eavesdropping, particularly when transmitting sensitive data over the network.
- Vulnerability Management: Regularly scanning and addressing security vulnerabilities in the API’s code, infrastructure, and dependencies to prevent potential exploits.
These security measures are essential for building trustworthy and reliable RESTful APIs with Python and Flask. They protect sensitive data from unauthorized access, ensuring compliance with data protection regulations and maintaining the trust of users. By implementing comprehensive security measures, developers can safeguard the integrity and confidentiality of data exchanged through their APIs.
Testing
In the context of building RESTful APIs with Python and Flask for efficient data exchange, testing plays a pivotal role in ensuring the reliability and correctness of the API. Automated tests provide a systematic approach to verifying the API’s functionality, reducing the risk of errors and ensuring consistent behavior.
- Unit Testing: Testing individual components or modules of the API in isolation, ensuring they function as expected.
- Integration Testing: Verifying the interactions between different components of the API, ensuring they work together seamlessly.
- Functional Testing: Evaluating the API’s overall functionality from a user’s perspective, ensuring it meets the intended requirements.
- Performance Testing: Assessing the API’s performance under varying loads, ensuring it can handle real-world usage patterns.
These testing facets collectively contribute to the robustness and reliability of RESTful APIs built with Python and Flask. They help identify and resolve issues early in the development process, reducing the likelihood of production failures and enhancing the overall quality of the API. By incorporating automated testing into their development workflow, developers can build APIs that are not only efficient in data exchange but also highly reliable and maintainable.
Documentation
Documentation plays a vital role in the context of building RESTful APIs with Python and Flask for efficient data exchange. Clear and comprehensive documentation enables developers, consumers, and maintainers to understand the API’s purpose, functionality, and usage, fostering seamless integration and adoption. It serves as a valuable resource for onboarding new users, reducing support queries, and promoting API adoption.
- API Definition and Structure: Documenting the API’s endpoints, request and response formats, error codes, and versioning information provides a structured overview of the API’s capabilities.
- Code Examples and Usage Guidelines: Including code snippets and detailed usage instructions empowers developers to quickly integrate the API into their applications, reducing development time and potential errors.
- Tutorials and How-to Guides: Providing step-by-step guides and tutorials helps users understand complex API features and workflows, enabling them to make the most of the API’s capabilities.
Well-documented RESTful APIs built with Python and Flask facilitate efficient data exchange by enabling seamless integration, reducing the learning curve for developers, and promoting consistent API usage. By providing clear and comprehensive documentation, API creators can empower users to leverage the API’s full potential, ultimately fostering a thriving ecosystem of data exchange and application development.
Performance Optimization
Performance optimization is a critical aspect of building RESTful APIs with Python and Flask for efficient data exchange. By implementing techniques like caching, pagination, and others, APIs can handle increasing data volumes and complex operations while maintaining fast response times and efficient resource utilization.
- Caching: Storing frequently requested data in memory to reduce database queries and improve response times.
- Pagination: Dividing large datasets into smaller pages to avoid sending excessive data in a single response, improving performance for API consumers with limited bandwidth.
- Content Delivery Networks (CDNs): Distributing API content across multiple servers located in different geographical regions, reducing latency and improving accessibility for global users.
- Load Balancing: Distributing incoming API requests across multiple servers to handle high traffic volumes, ensuring API availability and scalability.
These optimization techniques collectively contribute to enhancing the overall performance and responsiveness of RESTful APIs built with Python and Flask. By implementing these strategies, developers can create APIs that can efficiently exchange data, handle high traffic volumes, and provide a seamless user experience for API consumers.
FAQs on Building RESTful APIs with Python and Flask for Efficient Data Exchange
This FAQ section addresses common questions and provides additional insights into building RESTful APIs with Python and Flask for efficient data exchange.
Question 1: What are the key advantages of using Python and Flask for building RESTful APIs?
Python and Flask offer several advantages, including their ease of use, extensive library support for data manipulation and web development, and Flask’s lightweight and flexible nature, making it suitable for building scalable and efficient APIs.
Question 2: How does caching improve API performance?
Caching involves storing frequently requested data in memory, reducing the need for database queries and significantly improving response times. By leveraging caching mechanisms, APIs can handle increased traffic and maintain fast and consistent performance.
Question 3: What is the purpose of pagination in RESTful APIs?
Pagination divides large datasets into smaller, manageable pages. This technique prevents overwhelming API consumers with excessive data in a single response, enhances performance, and accommodates limited bandwidth scenarios.
Question 4: How can I handle authentication and authorization in my RESTful API?
To ensure data security and controlled access, implement authentication and authorization mechanisms. Authentication verifies user identity, while authorization determines their access privileges to specific API resources and operations.
Question 5: What are some best practices for writing efficient RESTful API endpoints?
Follow RESTful principles, use descriptive and consistent naming conventions for endpoints, handle errors gracefully, and consider using versioning to manage API evolution and maintain compatibility with existing clients.
Question 6: How can I monitor and troubleshoot my RESTful API?
Implement logging and monitoring mechanisms to track API performance, identify errors, and gather insights into usage patterns. This enables proactive issue detection and resolution, ensuring API reliability and availability.
These FAQs provide a deeper understanding of key aspects of building RESTful APIs with Python and Flask for efficient data exchange. The next section will delve into advanced topics and best practices for optimizing API performance and security.
Tips for Building RESTful APIs with Python and Flask for Efficient Data Exchange
This section provides practical tips and best practices for building efficient and effective RESTful APIs using Python and Flask. By implementing these tips, developers can enhance the performance, security, and maintainability of their APIs.
Tip 1: Utilize Caching Mechanisms: Implement caching to store frequently accessed data in memory, reducing database queries and improving response times.
Tip 2: Implement Pagination: Divide large datasets into smaller pages for API responses, optimizing performance for clients with limited bandwidth.
Tip 3: Leverage Content Delivery Networks (CDNs): Distribute API content across multiple servers globally to reduce latency and improve accessibility for users in different regions.
Tip 4: Employ Load Balancing: Distribute incoming API requests across multiple servers to handle high traffic volumes, ensuring API availability and scalability.
Tip 5: Utilize Data Validation: Implement input validation to ensure that data received by the API meets expected formats and constraints, improving data integrity.
Tip 6: Implement Authentication and Authorization: Secure your API by implementing authentication and authorization mechanisms to control user access to specific resources and operations.
Tip 7: Use Versioning for API Evolution: Implement API versioning to manage API changes over time, ensuring compatibility with existing clients while introducing new features.
Tip 8: Monitor and Troubleshoot Your API: Regularly monitor your API’s performance, log errors, and gather usage patterns to identify areas for improvement and resolve issues proactively.
By following these tips, developers can build robust, efficient, and secure RESTful APIs with Python and Flask, enabling seamless data exchange and enhancing the overall user experience.
These tips lay the foundation for the concluding section, which will delve into advanced topics and best practices for optimizing API performance, security, and maintainability.
Conclusion
In conclusion, building RESTful APIs with Python and Flask offers a powerful approach for efficient data exchange. This article explored various facets of this topic, emphasizing the significance of architecture, data modeling, routing, serialization, security, testing, documentation, and performance optimization. By implementing these concepts and best practices, developers can create robust and scalable APIs that meet the demands of modern applications and services.
Key takeaways from this article include:
- RESTful APIs built with Python and Flask provide structured and efficient data exchange mechanisms.
- Proper API design, data modeling, and security measures are crucial for ensuring data integrity and reliability.
- Performance optimizations, such as caching and pagination, enhance API responsiveness and scalability.
The ability to build RESTful APIs with Python and Flask empowers developers to create innovative data-driven solutions. These APIs facilitate seamless data exchange, enabling interoperability and unlocking new possibilities for application integration. As the demand for efficient data exchange continues to grow, mastering the art of building RESTful APIs with Python and Flask will become increasingly valuable for developers seeking to contribute to the future of data-centric applications and services.
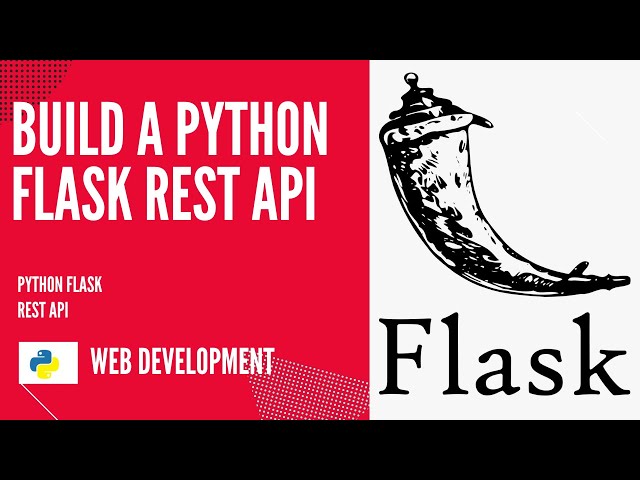