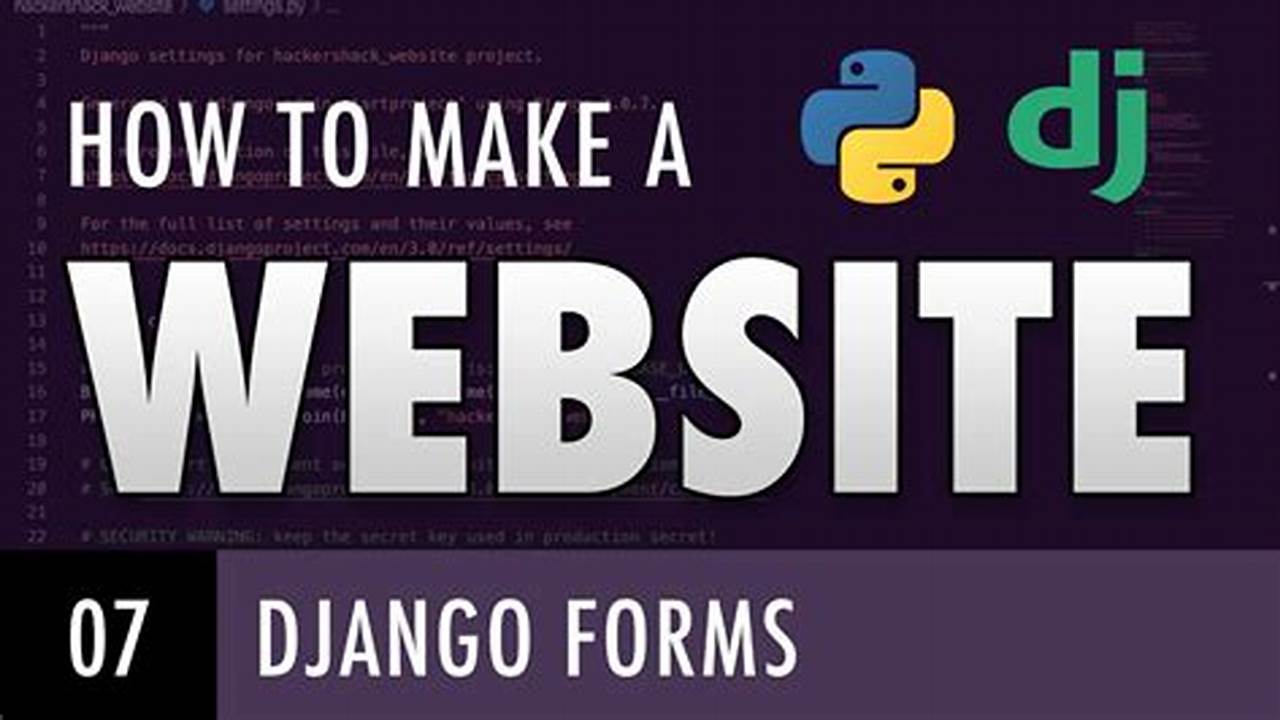
Building a Simple Web Application Using the Django Framework in Python refers to the process of creating a functional website or web application with the help of the Django web framework and the Python programming language.
This approach is widely used in web development due to Django’s user-friendly interface, extensive features, and ability to handle complex projects. It streamlines web application development by providing pre-built components, such as an object-relational mapping (ORM) system and an admin interface, which significantly reduces development time and effort.
The article delves into the key elements, advantages, and historical evolution of using Django with Python for web application development. It provides practical examples, discusses the benefits of using this combination, and explores the future prospects of this technology stack.
Building a Simple Web Application Using the Django Framework in Python
When building a web application using the Django framework in Python, several key aspects are crucial to consider. These aspects encompass various dimensions of web application development, ranging from technical components to design principles.
- Architecture: Layered structure for organizing code and improving maintainability.
- Models: Representation of data in the application, defining database structure.
- Views: Responsible for handling user requests and generating responses.
- Templates: HTML templates used to render dynamic content based on data.
- URL Routing: Mapping URLs to specific views for handling requests.
- Middleware: Intercepts requests and responses, providing additional functionality.
- Forms: User input handling, validation, and data processing.
- Admin Interface: Built-in interface for managing data and performing administrative tasks.
These aspects are interconnected and work together to create a robust and user-friendly web application. Understanding and effectively utilizing these aspects can significantly enhance the development process, ensuring code quality, maintainability, and a positive user experience.
Architecture
In the context of building a web application using the Django framework in Python, architecture plays a crucial role in ensuring code organization and maintainability. A well-structured architecture provides a clear and logical organization of the codebase, making it easier to understand, maintain, and extend.
Django’s architecture follows the Model-View-Template (MVT) design pattern, which separates the application into three distinct layers: models, views, and templates. This separation of concerns promotes code reusability, maintainability, and scalability. The model layer defines the data structures and business logic, the view layer handles user interactions and HTTP requests, and the template layer is responsible for rendering the user interface.
A layered architecture also facilitates collaboration and code ownership within a development team. Different team members can work on specific layers without affecting the others, leading to increased efficiency and reduced code conflicts. For example, a back-end developer can focus on the model and view layers, while a front-end developer can work on the template layer.
In summary, a layered architecture is a critical component of building a simple web application using the Django framework in Python. It provides a structured approach to code organization, promotes maintainability and scalability, and supports collaborative development efforts.
Models
In the context of building a simple web application using the Django framework in Python, models play a fundamental role in defining the structure and organization of data within the application. They act as blueprints for database tables, specifying the fields, data types, and relationships between different data entities.
- Database Schema: Models define the database schema by specifying the fields, data types, and constraints for each table. This ensures data integrity and consistency throughout the application.
- Object-Relational Mapping (ORM): Django’s ORM provides an abstraction layer between Python objects and database tables. Models serve as the Python representation of database tables, making it convenient to interact with data using Python code.
- Data Manipulation: Models provide methods for creating, retrieving, updating, and deleting data from the database. This simplifies data manipulation tasks and reduces the need for writing raw SQL queries.
- Data Validation: Models can specify validation rules to ensure that data meets certain criteria before being saved to the database. This helps maintain data quality and prevents invalid data from entering the system.
In summary, models are a crucial aspect of building a simple web application using the Django framework in Python. They provide a structured and efficient way to represent and manage data, ensuring data integrity, simplifying data manipulation, and reducing the need for writing raw SQL queries.
Views
In the context of building a simple web application using the Django framework in Python, views are a crucial component responsible for handling user requests and generating appropriate responses. The interaction between views and user requests is the cornerstone of web application functionality, enabling users to interact with the application and receive tailored responses.
When a user sends a request to the web application, Django routes the request to the appropriate view function based on the URL pattern matching. The view function then processes the request, interacts with the models to retrieve or update data, and generates a response. This response can be in various formats, such as HTML, JSON, or XML, depending on the application’s requirements.
Views play a critical role in determining the application’s behavior and the user experience. They define the logic for handling different types of requests, such as displaying a list of products, processing a form submission, or handling user authentication. Without views, the application would not be able to respond to user actions and fulfill its intended purpose.
Real-life examples of views in Django applications include:
- A view function that displays a list of blog posts on the home page.
- A view function that allows users to submit a contact form and sends an email to the website owner.
- A view function that handles user registration and authentication.
Understanding the role of views is essential for building effective and user-friendly web applications using Django. By creating well-structured and efficient views, developers can ensure that the application responds appropriately to user requests and delivers a seamless user experience.
Templates
In the context of building a simple web application using the Django framework in Python, templates play a pivotal role in generating dynamic and interactive web pages. They are HTML templates that define the structure and layout of the web pages, allowing developers to separate the presentation logic from the application logic. This separation of concerns promotes code reusability, maintainability, and the ability to easily update the application’s appearance without affecting its functionality.
Django’s templating system provides a powerful set of features for creating dynamic web pages. Templates can access data from models and views, enabling the display of real-time data and user-specific content. This dynamic nature is crucial for building web applications that respond to user interactions and display relevant information based on the user’s context.
Real-life examples of templates in Django applications include:
- Creating a template for the home page that displays a list of recent blog posts.
- Developing a template for a product detail page that displays product information and allows users to add the product to their cart.
- Designing a template for a user profile page that shows the user’s personal information and recent activity.
Understanding the role of templates is essential for building visually appealing and user-friendly web applications using Django. By effectively utilizing templates, developers can create dynamic and interactive web pages that adapt to the user’s needs and provide a seamless user experience.
URL Routing
In the context of building a simple web application using the Django framework in Python, URL routing plays a crucial role in directing user requests to the appropriate views for handling. It establishes a mapping between URLs and specific views, ensuring that the application responds appropriately to user actions and displays the intended content.
URL routing is a critical component of Django applications, as it determines how the application responds to different URLs. Without proper URL routing, the application would not be able to handle user requests effectively, leading to a poor user experience and potential security vulnerabilities.
Real-life examples of URL routing in Django applications include:
- Mapping the root URL (“/”) to a view that displays the home page.
- Mapping a URL like “/products/” to a view that lists all products in the database.
- Mapping a URL like “/products/create/” to a view that allows users to create a new product.
Understanding the importance of URL routing is essential for building robust and user-friendly web applications using Django. By effectively implementing URL routing, developers can ensure that the application responds correctly to user requests, displays the appropriate content, and provides a seamless user experience.
Middleware
Middleware plays a crucial role in building a simple web application using the Django framework in Python. It acts as a bridge between the web server and the Django application, intercepting HTTP requests and responses and providing additional functionality. This allows developers to enhance the application’s behavior and add custom functionality without modifying the core code.
- Session Management: Middleware can manage user sessions, storing and retrieving session data across multiple requests. This enables features such as shopping carts and personalized experiences.
- Authentication and Authorization: Middleware can handle user authentication and authorization, ensuring that only authorized users have access to specific parts of the application.
- Caching: Middleware can cache frequently accessed data, reducing the load on the database and improving application performance.
- Exception Handling: Middleware can intercept errors and exceptions, providing custom error handling and logging mechanisms.
By leveraging middleware, developers can extend the capabilities of their Django applications, add custom functionality, and improve the overall user experience. Middleware provides a powerful and flexible mechanism to enhance the application’s behavior and cater to specific business requirements.
Forms
Forms play a crucial role in building a simple web application using the Django framework in Python. They provide a standardized and efficient mechanism for collecting, validating, and processing user input, making them essential for tasks such as user registration, product purchases, and feedback collection.
- Data Collection: Forms allow users to submit data to the application, such as personal information, product orders, or survey responses. They are customizable to cater to various data collection requirements.
- Input Validation: Forms can validate user input to ensure the data entered is complete, correct, and in the expected format. This validation helps prevent errors, improve data quality, and reduce the need for manual data validation.
- Data Processing: Forms can process the submitted data to perform specific actions, such as creating user accounts, placing orders, or sending emails. This processing is essential for completing user-initiated tasks and driving the application’s functionality.
- User Interface: Forms provide a user-friendly interface for collecting user input. They can be customized to match the application’s design and incorporate elements like text fields, drop-down menus, and checkboxes to facilitate user interaction.
Effectively utilizing forms is crucial for building a user-friendly and efficient web application. They streamline data collection, ensure data integrity, and provide a consistent user experience. By leveraging Django’s built-in form handling capabilities and customizing forms to meet specific application requirements, developers can significantly enhance the overall user experience and streamline application development.
Admin Interface
In the context of building a simple web application using the Django framework in Python, the Admin Interface plays a crucial role in providing a user-friendly and efficient interface for managing data and performing administrative tasks. It allows authorized users to perform various operations essential for the maintenance and administration of the application’s data and functionality.
-
User Management:
The Admin Interface enables the creation, editing, and deletion of user accounts, including setting permissions and roles for different users. This is particularly useful for managing multiple users with varying levels of access to the application. -
Data Manipulation:
Through the Admin Interface, users can add, modify, and delete data in the application’s database. This allows for easy management of content, products, orders, or any other data models defined within the application. -
Content Management:
For applications with content-driven functionality, the Admin Interface provides a streamlined interface for managing content such as blog posts, articles, or pages. It allows for easy editing, previewing, and publishing of content without the need for technical expertise. -
System Configuration:
The Admin Interface often includes options for configuring system settings, such as site-wide parameters, email settings, or payment gateways. This enables administrators to customize the application’s behavior and functionality according to their specific requirements.
The Admin Interface in Django is a powerful tool that simplifies the management and administration of web applications. It provides a centralized and intuitive interface for authorized users to perform various tasks, ensuring the smooth operation and maintenance of the application.
Frequently Asked Questions on Building a Simple Web Application Using the Django Framework in Python
This FAQ section aims to clarify common questions and provide key insights related to building simple web applications using Django in Python. It addresses fundamental aspects, best practices, and potential challenges to guide developers effectively.
Question 1: What are the key benefits of using Django for web development?
Django offers a comprehensive set of features and tools that streamline web development. It provides a Model-View-Template (MVT) architecture, an Object-Relational Mapper (ORM), and a user-friendly admin interface, enabling rapid development and maintainable code.
Question 2: How do I choose the right database for my Django application?
Django supports various database systems, including PostgreSQL, MySQL, SQLite, and Oracle. The choice depends on factors such as scalability, performance requirements, and data size. PostgreSQL is a popular choice for its robustness and advanced features.
Question 3: What are the best practices for writing efficient Django views?
Django views should be concise, reusable, and handle errors gracefully. Utilize class-based views for complex logic, and favor generic views when possible. Optimize queries using prefetching and caching techniques to improve performance.
Question 4: How can I enhance the security of my Django application?
Django provides built-in security features, but additional measures are recommended. Implement user authentication and authorization, use secure protocols like HTTPS, and regularly update Django and its dependencies to mitigate vulnerabilities.
Question 5: What are the advantages of using a virtual environment for Django development?
Virtual environments isolate different Python installations and their dependencies for each project. This prevents conflicts, ensures reproducibility, and allows for easy management of specific application requirements.
Question 6: How can I deploy my Django application to a production server?
Various options are available for deploying Django applications, including using cloud platforms like Heroku or AWS Elastic Beanstalk. Choose a deployment method that aligns with your project’s requirements and ensures reliability and scalability.
In summary, building simple web applications using Django in Python involves understanding its key features, best practices, and potential challenges. By addressing these FAQs, developers can gain clarity on important aspects and make informed decisions during the development process.
As we delve deeper into the discussion, the next section will explore advanced topics related to Django web development, providing insights into optimizing performance, handling complex data, and integrating with external services.
Tips for Building a Simple Web Application Using the Django Framework in Python
This section provides practical tips to enhance your Django development experience and create efficient, user-friendly web applications. By following these tips, you can streamline your workflow, optimize performance, and deliver high-quality applications.
Tip 1: Utilize a Virtual Environment: Isolate your project’s Python environment and dependencies to prevent conflicts and ensure reproducibility.
Tip 2: Implement Class-Based Views: Enhance code organization and reusability by using class-based views for complex view logic.
Tip 3: Optimize Database Queries: Improve performance by leveraging Django’s query optimization techniques, such as prefetching and caching.
Tip 4: Secure Your Application: Implement robust security measures, including user authentication, authorization, and regular updates to mitigate vulnerabilities.
Tip 5: Leverage Built-in Django Features: Take advantage of Django’s comprehensive set of features, such as the admin interface, form handling, and template system.
Tip 6: Utilize Third-Party Packages: Enhance your application’s functionality by integrating with external Python packages for tasks like data visualization, social media integration, and payment processing.
Tip 7: Follow Best Practices: Adhere to established Django best practices, such as writing concise views, using generic views, and structuring your code effectively.
Tip 8: Test Your Code: Implement a comprehensive testing strategy to ensure the reliability and correctness of your application.
By incorporating these tips into your development process, you can build robust, scalable, and user-centric web applications using Django. These tips lay the foundation for the concluding section, which will explore advanced topics and best practices for handling complex data, optimizing performance, and integrating with external services.
Stay tuned for the final section, where we delve deeper into the art of crafting high-quality Django applications.
Conclusion
In summary, building a simple web application using the Django framework in Python involves a multifaceted approach that encompasses understanding core concepts, best practices, and advanced techniques. This article explored key aspects such as architecture, models, views, templates, URL routing, middleware, forms, and the admin interface, providing a solid foundation for Django development.
Two main points emerged from our discussion. Firstly, Django offers a comprehensive set of features and tools that streamline web development, making it an ideal choice for building scalable and maintainable applications. Secondly, adhering to best practices and leveraging advanced techniques is crucial for optimizing performance, enhancing security, and delivering a seamless user experience.
As we continue to advance in the realm of web development, Django remains a powerful and versatile framework that empowers developers to create robust, user-centric web applications. Embrace the principles outlined in this article, delve into further learning, and harness the potential of Django to bring your web development aspirations to life.
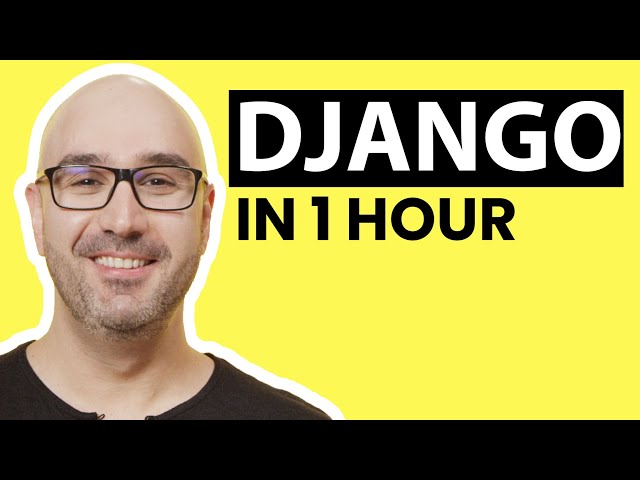